Welcome to the world of C programming! You’re not alone if you’ve ever been puzzled by the strange world of pointers. We set out to solve the mystery of pointers in C in this beginner-friendly adventure. In your computer’s memory, pointers serve as signposts, directing your software to certain areas. They improve the effectiveness of your code and let you work with data directly. Fear not, as we gradually explore these seemingly complicated creatures. By the time this journey is over, you will not only know what pointers are, but you will also recognize how powerful they can be to open up new possibilities for your C programming endeavors. Now let’s get started!
Table of Contents
What Are Pointers?
A pointer in C programming is a unique variable that holds the memory location of another variable. Pointers store the location (address) of a value in the computer’s memory rather than the value itself, as do regular variables.
Let’s break it down a bit:
- Variable: A data type-specific storage unit (such as a character, integer, etc.).
- Memory Address: A computer program’s variables are each assigned a specific address in the memory of the machine. For the variable, it serves as a home number.
- Pointer: Consider a pointer to be a variable’s GPS coordinate. It provides the location of the data in the computer’s memory rather than the actual data.
Now, let’s compare pointers with regular variables:
- Variables: They directly store data. For example, if you have an integer variable
num
with the value 10,num
holds that value.
int num = 10;
- Pointers: They hold a variable’s memory address. Although it doesn’t hold the value 10, a pointer ptr pointing to the location of num knows where to locate it.
int num = 10;
int *ptr = # // ptr now "points to" the memory address of num
To sum up, pointers let you deal with the memory locations where data is stored, enabling you to read and modify data indirectly.
Example:
#include <stdio.h>
int main() {
int num = 42;
int *ptr = #
printf("Value of num: %d\n", num);
printf("Address of num: %p\n", (void*)&num);
printf("Value through pointer: %d\n", *ptr);
return 0;
}
In this example, ptr
points to the memory address of num
. Using *ptr
retrieves the value stored at that address, which is the value of num
.
Declaration and Initialization Pointers in C
1. Syntax for Declaring Pointers:
Declaring a pointers in C programming entails providing the data type it will point to and naming it. Here is the general syntax:
data_type *pointer_name;
Here:
data_type
is the type of data the pointer will point to (e.g., int, float, char).*
indicates that we are declaring a pointer.pointer_name
is the chosen name for the pointer.
For example, to declare an integer pointer named ptr
:
int *ptr;
This declares a pointer called ptr
that can point to an integer.
2. Initializing Pointers with Addresses:
After declaring a pointer, you may initialize it by assigning it the memory address of a variable. This is a necessary step before manipulating or accessing data with the pointer. This is the syntax:
pointer_name = &variable;
Here:
pointer_name
is the name of the pointer.&variable
represents the memory address of the variable you want the pointer to point to.
For instance, let’s initialize the previously declared ptr
with the address of an integer variable num
:
int num = 10;
int *ptr; // Declaration
ptr = # // Initialization with the address of 'num'
Now, ptr
is pointing to the memory location where the value of num
is stored.
Combining both steps:
int *ptr; // Declaration
int num = 10;
ptr = # // Initialization with the address of 'num'
Following these actions, the num variable’s memory address is indicated by ptr, a pointer. To access or change the value kept in num, use ptr.
Pointer Arithmetic
Pointers in C may be used to carry out mathematical operations using pointer arithmetic. As you may know, pointers hold addresses in memory. In essence, we are shifting the pointer to a new position in memory when we do arithmetic operations on pointers.
Example:
int numbers[] = {1, 2, 3, 4, 5};
int *ptr = numbers; // Pointer pointing to the first element of the array
// Using pointer arithmetic to access elements
printf("%d\n", *ptr); // Output: 1
printf("%d\n", *(ptr+1)); // Output: 2
printf("%d\n", *(ptr+2)); // Output: 3
In this case, ptr+1 moves the pointer one element in the array, and ptr+2 moves it by two. Changing the memory location that a pointer points to is the fundamental idea of pointer arithmetic.
Incrementing and Decrementing Pointers in C:
In C, it’s standard practice to increment and decrement pointers, particularly when working with arrays. It involves moving the pointer to the memory address that is either next (increment) or previous (decrement).
Example:
int numbers[] = {10, 20, 30, 40, 50};
int *ptr = numbers; // Pointer pointing to the first element of the array
// Incrementing and decrementing pointers
ptr++; // Move to the next element
printf("%d\n", *ptr); // Output: 20
ptr--; // Move back to the previous element
printf("%d\n", *ptr); // Output: 10
In this example, ptr++
moves the pointer to the next element, and ptr--
moves it back to the previous element. The size of the data type to which the pointer points determines how many bytes the pointer is moved.
Dereferencing Pointers In C
Dereferencing a pointer is accessing or modifying the value stored at the memory address to which the pointer points. It’s similar to following a treasure map to discover the actual treasure.
Accessing Data through Pointers:
Imagine you have a pointer pointing to a variable:
int number = 42;
int *pointer = &number; // Pointer points to the address of 'number'
To access the value stored at that memory address (in this case, the value of ‘number’), you use the dereference operator *
:
int value = *pointer; // Dereferencing the pointer to get the value
Now, value
holds the value 42, which is the content of the memory location pointed to by pointer
.
Modifying Data through Pointers:
You may also change the contents at the memory address that a pointer points to by dereferencing. Suppose you wish to use the pointer to alter the value of ‘number’:
*pointer = 99; // Modifying the value through the pointer
Now, if you check the value of ‘number’, it will be 99:
printf("%d", number); // Output: 99
Dereferencing is important because it lets you work with data in an indirect manner. This is particularly effective when working with data structures, functions, and dynamic memory allocation. It helps you from repeating a lot of data in your software and enables you to handle data efficiently.
Arrays and Pointers
Pointers and arrays are closely related in C programming. You may basically think of an array name as a pointer to the array’s first element.
Array Name as a Pointer:
When you declare an array in C, the array name represents the address of the first element in memory. For example:
int numbers[5] = {1, 2, 3, 4, 5};
int *ptr = numbers; // 'numbers' is equivalent to '&numbers[0]'
Here, numbers
is essentially a pointer pointing to the first element (&numbers[0]
) of the array.
Array Elements Using Pointers:
You can access array elements using pointers by dereferencing them. For instance:
int firstElement = *ptr; // Dereferencing the pointer to get the value of the first element
int secondElement = *(ptr + 1); // Accessing the second element using pointer arithmetic
Pointer Arithmetic with Arrays:
In pointer arithmetic, one uses pointers to move between an array’s elements. It’s crucial to remember that pointer arithmetic considers the data type’s size.
1. Incrementing Pointers:
When you increment a pointer, it moves to the next element of the array. The pointer automatically adjusts based on the size of the data type. For example:
int *ptr = numbers; // Points to the first element
ptr++; // Moves to the next element
2. Array Access Using Pointer Arithmetic:
Pointer arithmetic allows you to access array elements using offsets. For instance:
int thirdElement = *(ptr + 2); // Accessing the third element using pointer arithmetic
This is equivalent to numbers[2]
.
Functions and Pointers
1. Passing Pointers to Functions:
In C, you are effectively giving a function the memory address of a variable when you send it a pointer. This enables the function to access and change the value stored in that memory address. Here’s an easy example:
#include <stdio.h>
// Function that takes a pointer as an argument
void modifyValue(int *ptr) {
// Dereferencing the pointer to access and modify the value
*ptr = 20;
}
int main() {
int number = 10;
// Passing the address of 'number' to the function
modifyValue(&number);
// 'number' has been modified by the function
printf("Modified value: %d\n", number);
return 0;
}
In this example, modifyValue
takes a pointer (int *ptr
) as an argument. The &number
in the main
function passes the memory address of the number
variable to modifyValue
. The function then modifies the value at that memory location to 20.
2. Modifying Values Using Pointers in Functions:
In C, when you pass a pointer to a function and modify the value through that pointer, the changes are reflected outside the function. This is because you are directly working with the memory location of the original variable. Here’s an example:
#include <stdio.h>
// Function that modifies the value through a pointer
void modifyValue(int *ptr) {
// Dereferencing the pointer to modify the value
*ptr = *ptr * 2;
}
int main() {
int number = 5;
// Passing the address of 'number' to the function
modifyValue(&number);
// 'number' has been modified by the function
printf("Modified value: %d\n", number);
return 0;
}
In this example, modifyValue
takes a pointer as an argument and doubles the value at that memory location. The main
function passes the address of number
to modifyValue
, and the modified value is then printed. The result is that number
is now 10.
Dynamic Memory Allocation in C
Sometimes, while working with C programming, you are unsure of the amount of data that will need to be stored in memory in advance. You can dynamically allocate memory in certain situations while your application is running. You may manage memory as needed with dynamic memory allocation, which is made possible by four essential features.
1. malloc
– Memory Allocation
malloc stands for “memory allocation.” It is used to dynamically allocate a specified number of bytes in memory.
int *ptr = (int*)malloc(4 * sizeof(int));
This example allocates memory to store four integers.
2. calloc
– Contiguous Allocation
calloc
stands for “contiguous allocation.” It allocates memory for an array of elements, initializing all bytes to zero.
int *ptr = (int*)calloc(4, sizeof(int));
This example allocates memory for an array of four integers, initializing them to zero.
3. realloc
– Reallocation
realloc
stands for “reallocation.” It is used to change the size of a previously allocated block of memory.
int *ptr = (int*)malloc(4 * sizeof(int));
ptr = (int*)realloc(ptr, 8 * sizeof(int));
This example reallocates memory to store eight integers instead of four.
4. free
– Freeing Memory
free
is used to deallocate the memory previously allocated by malloc
, calloc
, or realloc
.
free(ptr);
This example frees the memory allocated for the dynamic array.
Pointers vs. Arrays
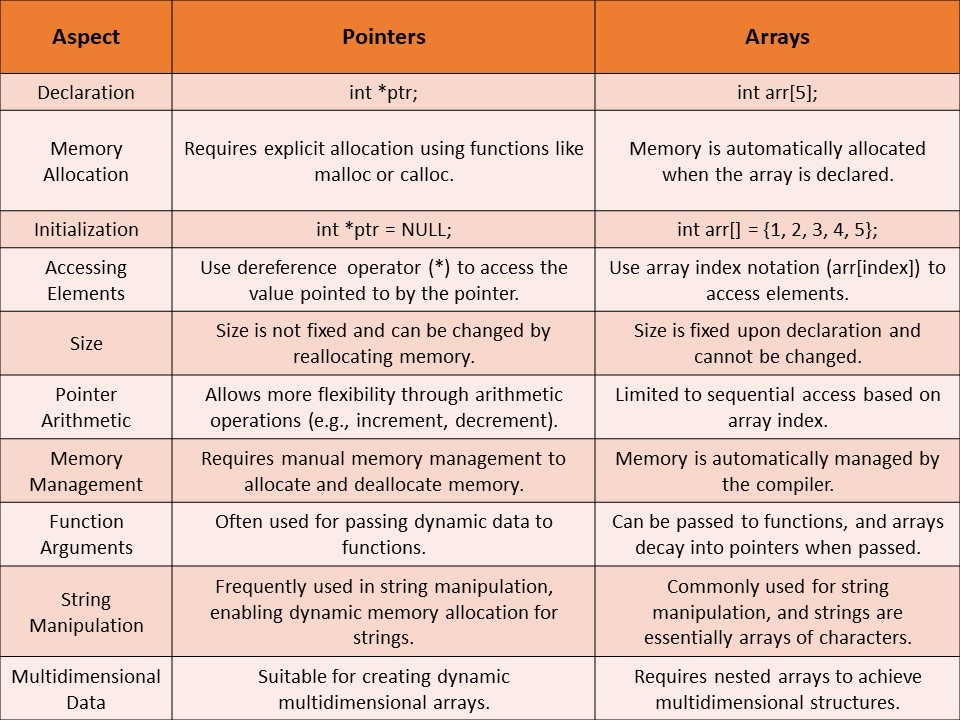