PHP, or Hypertext Preprocessor, is a robust programming language primarily used in web development. It enables dynamic web pages by allowing users to interact with online pages in real-time. Consider it the unseen magic that brings webpages to life.
Since PHP syntax is the building block of any PHP code, it is essential knowledge for beginners to learn. Like grammar in spoken languages, syntax refers to the rules and structure of the language. It’s similar to building a home without understanding how to use a hammer and nails.
Understanding PHP syntax allows you to develop code that performs specific functions like form data processing, database interaction, and dynamic content generation. With the help of this information, you may develop dynamic and interactive web applications that improve user experience and expand the functionality of websites.
PHP syntax is essentially the language that tells the computer what to do and how to do that, according to your instructions. Thus, learning PHP syntax is the first step for novice programmers starting their web development path towards becoming skilled in developing dynamic and captivating online apps.
Table of Contents
What is PHP? Why is it important?
PHP stands for Hypertext Preprocessor. It is a programming language used for developing dynamic web pages. When you visit a website, your browser requests the server, which uses PHP to process the request and build the web page you see.
PHP is known as a server-side scripting language since it operates on a web server rather than in your browser. The PHP code on the server interprets the user’s actions on the website and sends the appropriate response to display on their browser.
PHP functions as the chef in a website’s restaurant, receiving orders (requests), preparing ingredients (data processing), and giving visitors delicious dishes (dynamic web pages). Its function as a server-side scripting language is essential for adding features and interaction to webpages.
Key features and advantages of using PHP
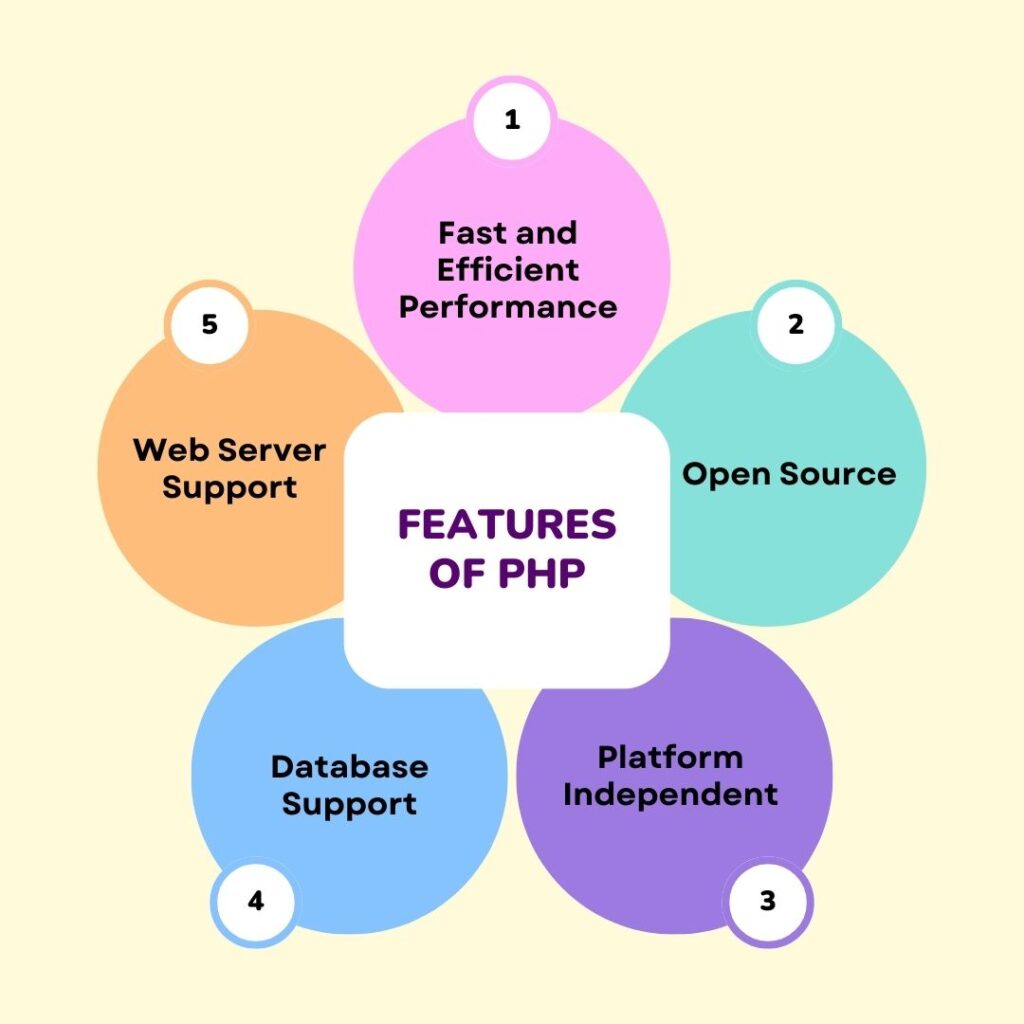
- Ease of Use: PHP is simple to learn and use, particularly for beginners. Its syntax is similar to other languages like C and Perl, making it understandable to many programmers.
- Versatility: Because developers can easily combine PHP code with standard HTML text, they directly insert PHP into HTML. It makes it simple to develop dynamic web pages that change content based on user interactions or database queries.
- Open-Source: PHP is an excellent programming language that is both free and open-source. It has a large community of developers and plenty of online resources. With so many people using and contributing to it, PHP is advancing to cater to the requirements of contemporary web development.
- Platform Independence: PHP is a versatile option for web development since it operates on many operating systems, including Windows, macOS, and Linux.
Popular use cases for PHP in web development
- Dynamic Websites: PHP is frequently used to build dynamic websites that, in response to user input, database queries, or other outside sources, dynamically generate content.
- Content Management Systems (CMS): Many well-known CMS platforms, including WordPress, Joomla, and Drupal, are developed with PHP. These systems make it simple for people to maintain and produce content for websites without requiring a lot of technical expertise.
- E-commerce Websites: PHP is frequently used to create online stores and e-commerce websites that let companies safely offer goods and services to customers online.
- Web Applications: Many web applications, such as online booking systems, forums, and social media platforms, may be created with PHP.
Understanding PHP Tags
Writing PHP code requires an understanding of PHP tags. PHP tags indicate the start and finish of PHP code inside a document. PHP tags are generally of two types:
1. Standard PHP Tags:
- PHP tags consist of <?php…?>.
- The server’s PHP engine executes any code written between PHP tags.
- It is the most popular method for embedding PHP code into HTML text.
Example:
<?php
echo "Hello, world!";
?>
2. Short Echo Tags:
- PHP’s short echo tags provide a shortcut for echoing output.
- They consist of <?=…?>.
- These tags commonly output a single variable or expression from within HTML markup.
Example:
<p>Welcome, <?= $username ?></p>
Using PHP tags correctly ensures the smooth execution of your PHP code. Use <\?php…?> tags rather than the less portable PHP short tags (\?…?>) to optimise portability. Servers often suppress these short tags due to security concerns.
Variables, Data Types and Constants in PHP
Variables in PHP are similar to data storage containers. They have values and names. Here is how a variable is declared:
$name = "John";
In this example, $name
is the variable, and “John” is its value.
Data types specify the sort of data a variable can store. Numerous data kinds, including texts, integers, floats, Booleans, arrays, and more, are supported by PHP. As an example:
$age = 25; // integer
$height = 5.11; // float
$isStudent = true; // boolean
Constants and variables are alike in the sense that they store values. Constants are fixed values that remain unchanged throughout the program.
define("PI", 3.14);
echo PI; // Outputs: 3.14
Statements and Expressions in PHP Code
- Statements in PHP are instructions that carry out operations, including assigning values for variables, calling functions, or controlling programme flow.
- Expressions are collections of variables, values, operators, and function calls that produce a single value. An expression to calculate the sum of two variables is $sum = $num1 + $num2;
Comments in PHP
- PHP code can have comments added to it to provide explanations. The PHP engine ignores these comments.
- PHP single-line comments are placed at the beginning of the line and terminated with either // or #.
- Multi-line comments are delimited by /* and */ and can span several lines.
<?php
// This is a single-line comment in PHP
/*
This is a multi-line comment
spanning multiple lines in PHP
*/
$name = "John"; // Assigning a value to the $name variable
echo "Hello, $name!"; // Echoing a greeting message
?>
By understanding these basic concepts of PHP syntax, you can start writing simple PHP scripts and gradually build your skills as a PHP programmer.