PHP, a popular scripting language for web development, has several techniques for creating output for display on web pages. Two important functions stand out: echo and print in PHP. While both serve the same purpose of displaying things to users, they have small but significant differences.
While many PHP developers use echo and print interchangeably, knowing their slight variations might help you write more effective and efficient code. Echo is a PHP language construct rather than a function, providing it slightly more speed and flexibility. It is commonly used to output variables and strings directly into HTML text.
However, print is a PHP function that provides a more standardized method of text output. It returns a value (1), making it useful in expressions. But in some situations, even small behavioral variations can have an impact on performance.
In this tutorial, we’ll look at the syntax, behavior, and recommended practices for echo and print in PHP. Understanding these differences can help developers decide when to apply each technique, which will improve the readability and efficiency of their PHP code.
Table of Contents
Echo in PHP
Echo is a PHP language construct, so it does not require brackets when used. It is one of the most used ways to output data in PHP.
Echo is that awesome friend who’s never afraid to speak out. It does not return a value; instead, it simply says anything you tell it to without thinking twice. It works wonderfully for showing HTML elements, variables, and strings straight on your website.
Here’s a short example. Assume you wish to greet your website visitors with a welcoming message. You would write:
echo "Welcome to our website!";
Characteristics and Behavior:
- Echo doesn’t have a return value. It just outputs one or more strings.
- It can print several parameters separated by commas.
- Echo is faster than print because it does not return a value.
- It can be used with or without brackets; however, the latter is more usual.
Examples
Example 1: Outputting a simple string
echo "Hello, world!";
Example 2: Outputting multiple strings
echo "Hello", " ", "world!";
Example 3: Outputting variables
$name = "John";
echo "Hello, $name!";
Example 4: Combining echo with HTML
echo "<h1>Welcome to my website</h1>";
Echo is simple and versatile, making it a popular choice for outputting data in PHP scripts. It is ideal for quickly showing content without trouble.
Print in PHP
To output data, use the print statement in PHP. Its syntax is simple: print, followed by the data to print, contained in parenthesis if you’re printing more than one expression.
Characteristics and Behavior:
- Print has a return value, in contrast to echo. When a return value is required, it can be helpful that it always returns 1.
- Print, like echo, transmits output straight to the client or browser, bypassing any buffering.
- Print only accepts a single argument, even though it may be a complex expression.
Examples Illustrating Print Usage
Example 1: Basic Text Output
print "This is a simple text.";
In this example, print
simply outputs the text “This is a simple text.” to the screen.
Example 2: Outputting Variables
$name = "Alice";
print ("Hello, " . $name . "!");
In this case, we’re using print
to output HTML content stored in a variable. This could be useful when generating dynamic HTML content within PHP.
Example 4: Concatenating with Print
$age = 30;
print "I am " . $age . " years old.";
Here, we’re concatenating the value of the $age
variable with some text before printing it out. The output would be “I am 30 years old.”
Example 5: Using Print in a Function
function greet($name) {
print "Hello, " . $name . "!";
}
greet("Emily");
In this example, we define a function greet()
that takes a name as a parameter and prints out a greeting message using print
. Then, we call this function with the name “Emily”, resulting in the output “Hello, Emily!”.
Key Differences between echo and print in PHP
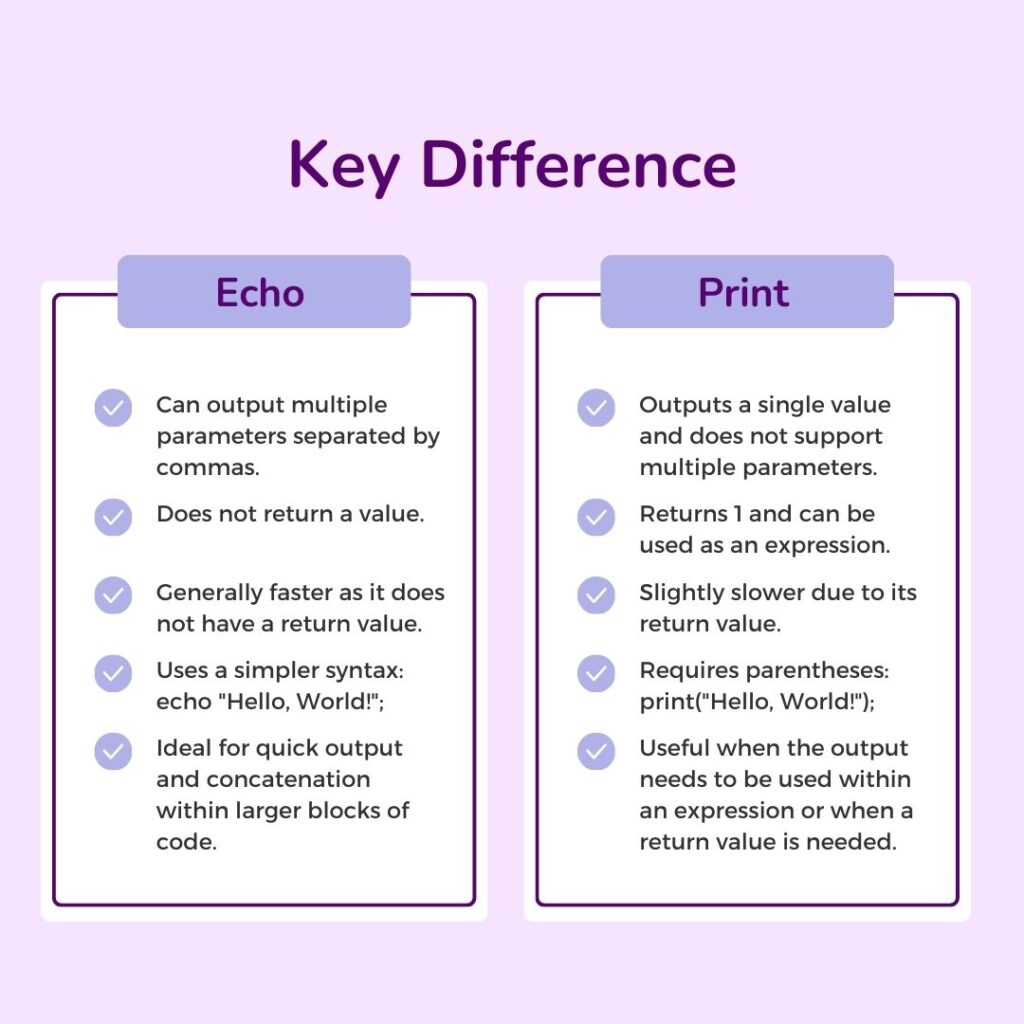
Developers love Echo because of its efficiency and simplicity; it doesn’t require brackets and is simple. On the other hand, print returns a value but is slightly slower because of its function-like behavior.
Consider your project’s setting and requirements before choosing between echo and print. Echo is the preferred option for fast outputs or concatenating strings. It is simple and lightweight. Print might be a better option, however, if you need to evaluate an expression or need a return value.