PHP operators are fundamental programming tools that enable developers to do mathematical calculations, comparisons, and logical operations. Composing PHP code that is both effective and efficient requires an understanding of these operators. A variety of PHP operators, such as arithmetic, assignment, comparison, logic, string, increment and decrement, ternary, and null coalescing operators, will be covered in this tutorial. By the end of this guide, you’ll understand how these operators work and how to use them to create cleaner, simpler PHP code. Let’s get started!
Table of Contents
What are PHP Operators?
In simple terms, operators in PHP are special symbols or keywords that allow you to perform different actions on variables and values. These actions can include arithmetic operations like addition and subtraction, comparison of values, logical operations, and more.
Arithmetic Operators
Arithmetic operators in PHP are fundamental tools that enable you to perform mathematical calculations within your code. They consist of modulus (%), division (/), multiplication (*), subtraction (-), and addition (+).
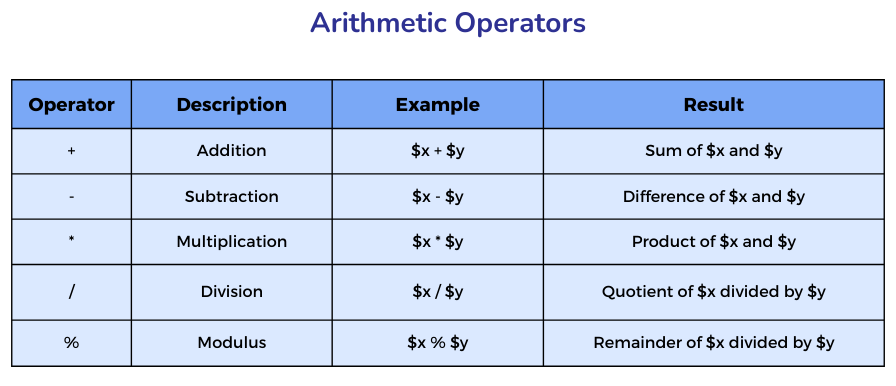
Example :
<?php
// Define variables
$x = 10;
$y = 3;
// Addition
$sum = $x + $y; // 10 + 3 = 13
// Subtraction
$diff = $x - $y; // 10 - 3 = 7
// Multiplication
$product = $x * $y; // 10 * 3 = 30
// Division
$quotient = $x / $y; // 10 / 3 = 3.333...
// Modulus
$remainder = $x % $y; // 10 % 3 = 1
// Output results
echo "Sum: " . $sum . "<br>";
echo "Difference: " . $diff . "<br>";
echo "Product: " . $product . "<br>";
echo "Quotient: " . $quotient . "<br>";
echo "Remainder: " . $remainder . "<br>";
?>
Output :
Sum: 13
Difference: 7
Product: 30
Quotient: 3.3333333333333
Remainder: 1
Assignment Operators
The assignment operators in PHP are used to assign values to variables. They can not only assign variables, but also conduct arithmetic operations simultaneously, making coding more simple and efficient.
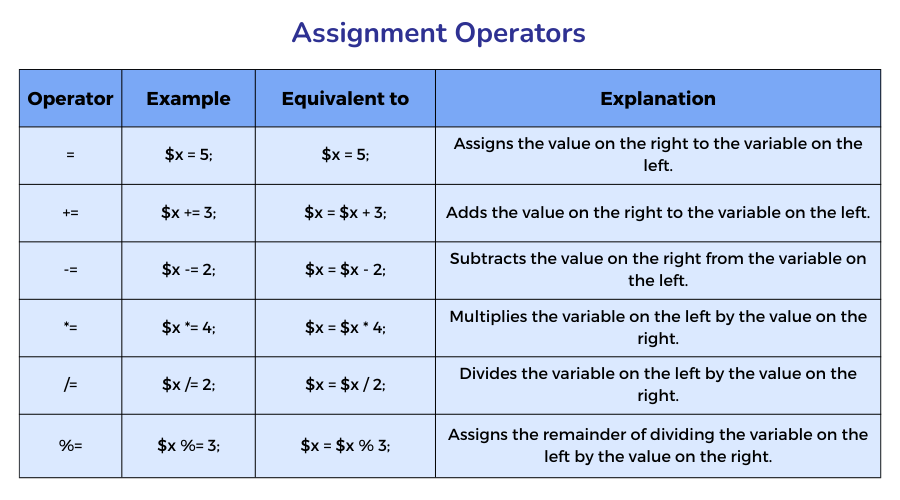
Example :
<?php
$x = 10;
// Assignment (=) Operator
$assignment = $x; // $assignment will be assigned the value of $x
echo "Assignment: " . $assignment . "<br>";
// Addition Assignment (+=) Operator
$x += 3; // Equivalent to: $x = $x + 3;
echo "Addition Assignment: " . $x . "<br>";
// Subtraction Assignment (-=) Operator
$x -= 2; // Equivalent to: $x = $x - 2;
echo "Subtraction Assignment: " . $x . "<br>";
// Multiplication Assignment (*=) Operator
$x *= 4; // Equivalent to: $x = $x * 4;
echo "Multiplication Assignment: " . $x . "<br>";
// Division Assignment (/=) Operator
$x /= 2; // Equivalent to: $x = $x / 2;
echo "Division Assignment: " . $x . "<br>";
// Modulus Assignment (%=) Operator
$x %= 3; // Equivalent to: $x = $x % 3;
echo "Modulus Assignment: " . $x . "<br>";
?>
Output :
Assignment: 10
Addition Assignment: 13
Subtraction Assignment: 11
Multiplication Assignment: 44
Division Assignment: 22
Modulus Assignment: 1
Comparison Operators
Comparison operators in PHP are used to compare two values and determine their relationship.
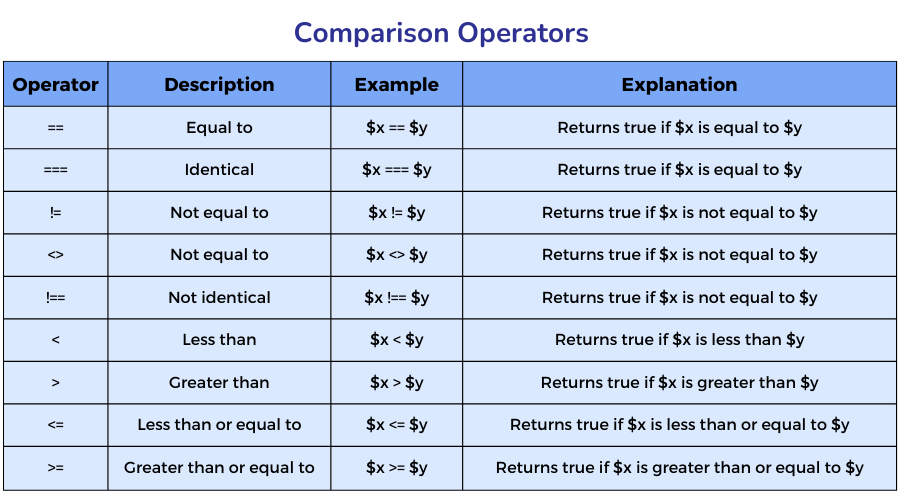
Example :
<?php
// Define variables
$x = 10;
$y = 5;
// Equal to
echo "Equal to (==): ";
var_dump($x == $y);
echo "<br>";
// Identical
echo "Identical (===): ";
var_dump($x === $y);
echo "<br>";
// Not equal to
echo "Not equal to (!=): ";
var_dump($x != $y);
echo "<br>";
// Not equal to (alternative)
echo "Not equal to (<>): ";
var_dump($x <> $y);
echo "<br>";
// Not identical
echo "Not identical (!==): ";
var_dump($x !== $y);
echo "<br>";
// Less than
echo "Less than (<): ";
var_dump($x < $y);
echo "<br>";
// Greater than
echo "Greater than (>): ";
var_dump($x > $y);
echo "<br>";
// Less than or equal to
echo "Less than or equal to (<=): ";
var_dump($x <= $y);
echo "<br>";
// Greater than or equal to
echo "Greater than or equal to (>=): ";
var_dump($x >= $y);
echo "<br>";
?>
Output :
Equal to (==): bool(false)
Identical (===): bool(false)
Not equal to (!=): bool(true)
Not equal to (<>): bool(true)
Not identical (!==): bool(true)
Less than (<): bool(false)
Greater than (>): bool(true)
Less than or equal to (<=): bool(false)
Greater than or equal to (>=): bool(true)
Logical Operators
Logical operators in PHP are used to perform logical operations on one or more expressions.
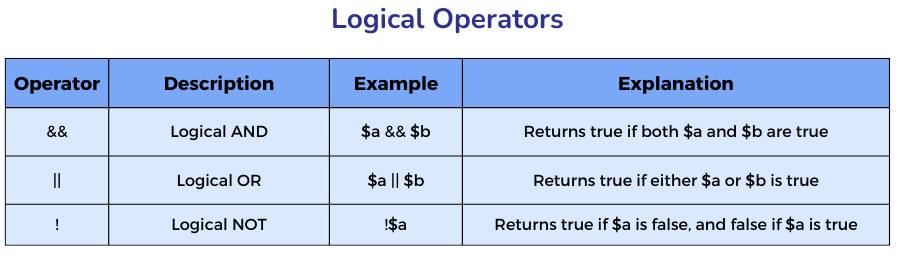
Example :
<?php
$a = true;
$b = false;
$result_and = $a && $b;
$result_or = $a || $b;
$result_not_a = !$a;
$result_not_b = !$b;
echo "Result of \$a && \$b: " . ($result_and ? 'true' : 'false') . "\n";
echo "Result of \$a || \$b: " . ($result_or ? 'true' : 'false') . "\n";
echo "Result of !\$a: " . ($result_not_a ? 'true' : 'false') . "\n";
echo "Result of !\$b: " . ($result_not_b ? 'true' : 'false') . "\n";
?>
Output :
Result of $a && $b: false
Result of $a || $b: true
Result of !$a: false
Result of !$b: true
String Operators
The string concatenation operator (.
) in PHP is used to join two or more strings together, creating a single string. It allows you to combine strings to form longer strings.
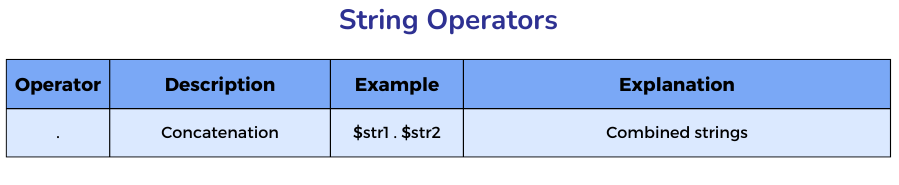
Example :
<?php
// Define strings
$str1 = "Hello";
$str2 = "World";
// Concatenation
$result = $str1 . " " . $str2;
echo "Concatenated String: " . $result;
?>
Output :
Concatenated String: Hello World
Increment and Decrement Operators
Increment and decrement operators in PHP are used to increase or decrease the value of a variable by one. In PHP, the increment operator (++) adds 1 to the value of a variable, while the decrement operator (–) subtracts 1 from the value of a variable.
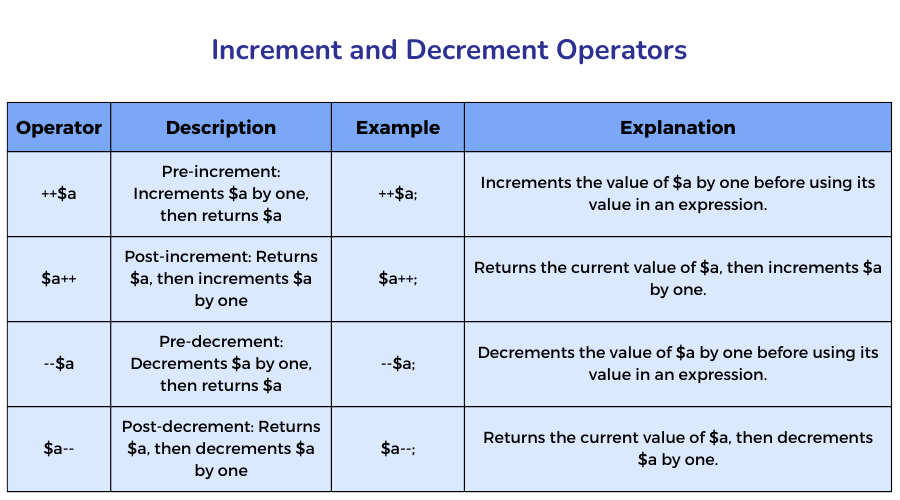
Example :
<?php
$a = 5;
echo "Initial value of \$a: " . $a . "<br>";
echo "Pre-increment: " . ++$a . "<br>"; // Pre-increment
echo "Value of \$a after pre-increment: " . $a . "<br>";
echo "Post-increment: " . $a++ . "<br>"; // Post-increment
echo "Value of \$a after post-increment: " . $a . "<br>";
echo "Pre-decrement: " . --$a . "<br>"; // Pre-decrement
echo "Value of \$a after pre-decrement: " . $a . "<br>";
echo "Post-decrement: " . $a-- . "<br>"; // Post-decrement
echo "Value of \$a after post-decrement: " . $a . "<br>";
?>
Output :
Initial value of $a: 5
Pre-increment: 6
Value of $a after pre-increment: 6
Post-increment: 6
Value of $a after post-increment: 7
Pre-decrement: 6
Value of $a after pre-decrement: 5
Post-decrement: 5
Value of $a after post-decrement: 4
Conditional Assignment Operators
Conditional assignment operators, also known as the shorthand assignment operators, are a set of operators in programming languages that combine assignment and conditional statements into a single concise expression. These operators allow you to assign a value to a variable based on a condition without the need for an explicit if-else statement.
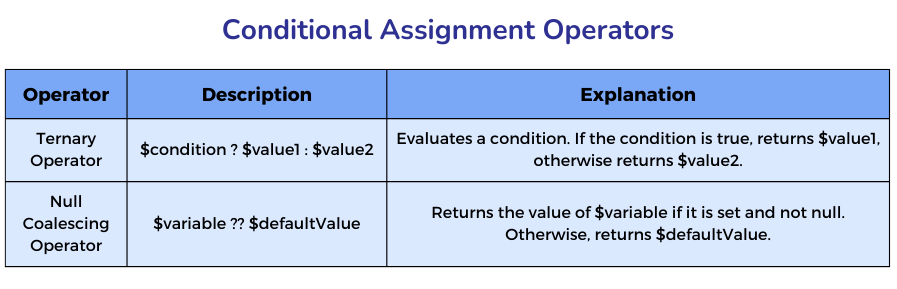
Example :
<?php
// Example demonstrating Ternary and Null Coalescing operators in PHP
// Ternary Operator
$age = 20;
$message = ($age >= 18) ? "You are an adult" : "You are a minor";
echo $message . PHP_EOL;
// Null Coalescing Operator
$username = isset($_GET['username']) ? $_GET['username'] : 'Guest';
echo "Welcome, $username" . PHP_EOL;
// Using Null Coalescing Operator with Ternary Operator
$user = $_GET['user'] ?? 'Guest';
$status = ($user === 'Guest') ? 'Not logged in' : 'Logged in';
echo "User status: $status" . PHP_EOL;
?>
Output :
You are an adult
Welcome, Guest
User status: Not logged in
Bitwise Operators
Bitwise operators in PHP are special operators that manipulate the individual bits of integers. In contrast to logical operators, which work with values that are either true or false (1 or 0), bitwise operators operate on the binary representation of integers, performing operations at the binary level.
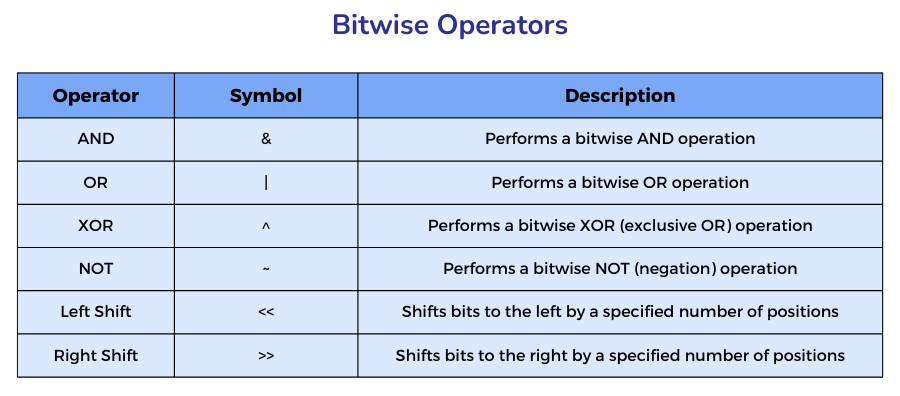
Example :
<?php
$a = 10; // Binary: 1010
$b = 6; // Binary: 0110
// Bitwise AND
$result_and = $a & $b; // Result: 0010 (2 in decimal)
// Bitwise OR
$result_or = $a | $b; // Result: 1110 (14 in decimal)
// Bitwise XOR
$result_xor = $a ^ $b; // Result: 1100 (12 in decimal)
// Bitwise NOT
$result_not_a = ~$a; // Result: 11111111111111111111111111110101 (-11 in decimal)
$result_not_b = ~$b; // Result: 11111111111111111111111111111001 (-7 in decimal)
// Left Shift
$result_left_shift = $a << 1; // Result: 10100 (20 in decimal)
// Right Shift
$result_right_shift = $a >> 1; // Result: 0101 (5 in decimal)
// Output
echo "Bitwise AND: $result_and\n";
echo "Bitwise OR: $result_or\n";
echo "Bitwise XOR: $result_xor\n";
echo "Bitwise NOT of \$a: $result_not_a\n";
echo "Bitwise NOT of \$b: $result_not_b\n";
echo "Left Shift of \$a: $result_left_shift\n";
echo "Right Shift of \$a: $result_right_shift\n";
?>
Output :
Bitwise AND: 2
Bitwise OR: 14
Bitwise XOR: 12
Bitwise NOT of $a: -11
Bitwise NOT of $b: -7
Left Shift of $a: 20
Right Shift of $a: 5
Array Operators
Array operators in PHP are used to perform operations specifically on arrays. These operators provide functionality for combining, comparing, and checking arrays.
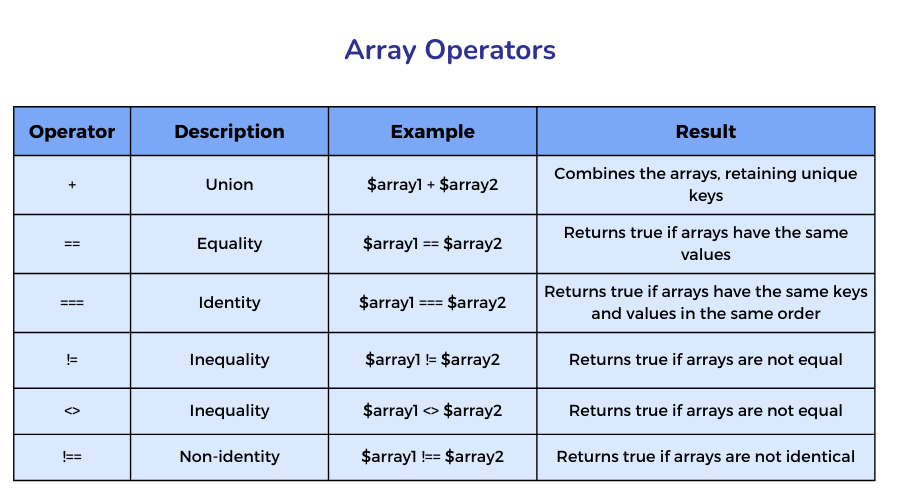
Example :
<?php
// Define two arrays
$array1 = array("a" => "apple", "b" => "banana");
$array2 = array("b" => "banana", "c" => "cherry");
// Union Operator (+)
$result_union = $array1 + $array2;
print_r($result_union);
// Equality Operator (==)
$result_equal = $array1 == $array2;
echo "Equality: ";
var_dump($result_equal);
// Identity Operator (===)
$result_identical = $array1 === $array2;
echo "Identity: ";
var_dump($result_identical);
// Inequality Operator (!=)
$result_inequality = $array1 != $array2;
echo "Inequality: ";
var_dump($result_inequality);
// Inequality Operator (<>)
$result_inequality2 = $array1 <> $array2;
echo "Inequality (<>): ";
var_dump($result_inequality2);
// Non-identity Operator (!==)
$result_non_identical = $array1 !== $array2;
echo "Non-identity: ";
var_dump($result_non_identical);
?>
Output :
Array
(
[a] => apple
[b] => banana
[c] => cherry
)
Equality: bool(false)
Identity: bool(false)
Inequality: bool(true)
Inequality (<>): bool(true)
Non-identity: bool(true)