Functions are crucial building blocks in C programming, acting as modular units to perform specified tasks. Understanding the various types and applications is critical for any aspiring programmer. In this article, we will look at C programming functions, breaking them down into different categories and seeing how they are used in real-world applications. Whether you’re a beginner learning the fundamentals or an experienced coder trying to improve your skills, this detailed study will give you with useful insights into the role and importance of functions in C programming. So, let’s go on the journey to discover the wonders of C functions.
Table of Contents
What are Functions?
In C programming, functions are small, specialised tools that help us in organising our code and performing certain tasks. Consider them as mini-programs within our main programme. They have names, and we can employ them to accomplish specific tasks.
A function in C is a part of code that performs a specified task. We refer to it by its name, and it is capable of accepting inputs, carrying out operations, and producing outputs for us. A function, like a recipe in a cookbook, instructs the computer on how to complete a specific activity.
Importance of Functions in C Programming
Functions are crucial in C programming for a few reasons:
- Organization: They help keep our code organized and manageable. Instead of having all of our code in one large block, we may divide it into smaller, more manageable bits.
- Reusability: Once we’ve developed a function to perform something, we can call it repeatedly throughout our programme without having to recreate the same code. Time is saved, and our programmes operate more smoothly as a result.
- Modularity: Functions enable us to break our programme into smaller, self-contained units. Our code can be easier to read and maintain if each function is dedicated to a single task.
Types of Functions in C Programming
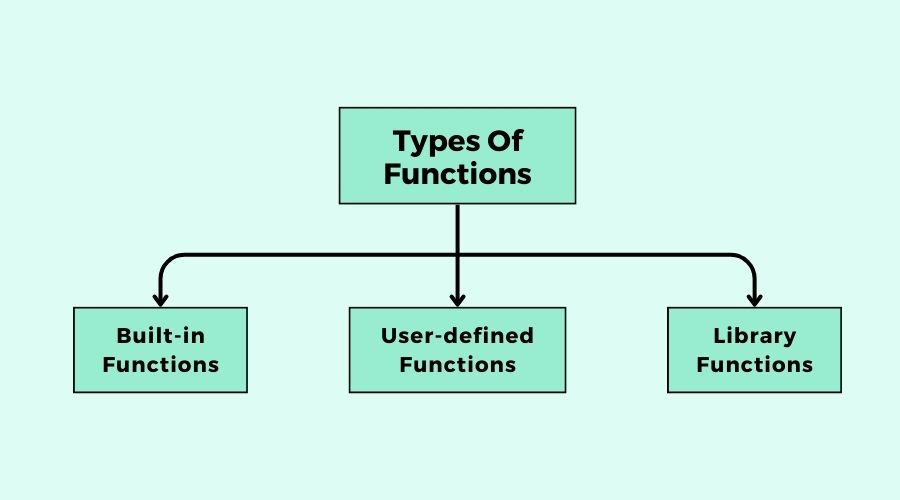
Built-in Functions:
Built-in functions are pre-defined in the C programming language. These functions are ready to use and execute specific tasks. Their functions include input/output operations, memory management, mathematical computations, and more. They are included in the C standard library.
Examples include printf()
and scanf()
, which are used for printing to the screen and taking input from the user, respectively.
User-defined Functions:
User-defined functions are functions created by the programmer in response to special requirements. These functions enable the programmer to divide a programme into smaller, more manageable chunks, improving code organisation, readability, and reuse. User-defined functions can execute any desired task and can be called repeatedly from within the programme.
For example, you might create a function called calculateArea()
to compute the area of a shape.
Library Functions:
Library functions are those provided by external libraries in C programming. These libraries contain a set of functions used for a variety of applications, including mathematical calculations, string manipulation, input/output activities, and so on. In order for programmers to utilise library functions, their programmes must have the necessary header files.
Examples include functions from the math.h
library, like sqrt()
for square root calculation and pow()
for exponentiation.
Built-in Functions
The C programming language has pre-defined functions that are referred to as built-in functions or standard functions. These functions can be used in any C programme without the programmer having to explicitly declare or define them. Built-in functions have specific uses and are intended to accomplish typical tasks effectively.
Examples:
- printf(): The printf() function displays formatted output to the screen. It allows you print text, variables, and other data in a predefined format.
int num = 10;
printf("The value of num is %d\n", num);
This will print “The value of num is 10” on the screen.
- scanf(): The function scanf() is used to read keyboard input from the user. It enables you to store data in variables and receive input in a predetermined format.
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
This will prompt the user to enter a number, read the input, and then print the entered number on the screen.
Usage and Applications:
Built-in functions are extensively used in C programming for various purposes, including:
- Input and Output Operations: Functions like printf() and scanf() are frequently used to display output to the user and read input from the user, respectively.
- Mathematical Operations: Built-in functions like sqrt(), pow(), sin(), cos(), and so on are used to execute mathematical computations.
- String Manipulation: You can manipulate strings using functions like strcpy(), strcat(), strlen(), and others.
- Memory Management: Malloc(), calloc(), realloc(), free(), and other functions are used for dynamic memory allocation and deallocation.
- File Operations: File handling operations are performed by using functions such as fopen(), fclose(), fread(), fwrite(), etc.
- Time and Date Functions: Time(), localtime(), strftime(), and other functions are used to manipulate dates and times.
User-defined Functions
User-defined functions are custom functions developed by the programmer to carry out specified duties within a C programme. They enable you to divide your programme into smaller, more manageable pieces, making it easier to understand, debug, and maintain.
Definition and Syntax:
A user-defined function is a piece of code that performs a specific function and can be called from anywhere in the programme.
The syntax for defining a user-defined function in C programming language is as follows:
return_type function_name(parameters) {
// Function body
// Code to perform the task
// Optional: return statement if the function returns a value
}
return_type
: Specifies the data type of the value that the function returns (e.g.,int
,float
,void
).function_name
: Name of the function that you choose.parameters
: Input values (arguments) that the function can accept (optional).function_body
: The block of code that performs the task of the function.
Parameters and Return Types
Parameters: Variables declared in the function declaration. They serve as placeholders for values given to the function when it is executed. A function can have zero or more parameters; parameters are optional.
Return Types: The return type indicates what type of value the function will return after execution. Any valid data type in C programming is acceptable, such as void, char, float, and int. If the function returns no value, the return type is set to void.
Examples:
Simple Functions: A simple function can accept parameters and return a value while carrying out a specific operation. For example:
// Function to add two numbers
int add(int num1, int num2) {
return num1 + num2;
}
Recursive Functions: A function that calls itself directly or indirectly is called a recursive function. It is helpful in resolving issues that can be divided into more manageable, related sub issues. For example:
// Factorial function using recursion
int factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
Advantages of User-defined Functions
- Modularity: Dividing a programme into smaller functions makes it more understandable and manageable.
- Reusability: Once defined, a function can be called from many parts of the programme, reducing code duplication.
- Easy Debugging: Isolating certain tasks within functions makes it easier to find and solve errors.
- Improved Readability: Well-named functions with clear meanings make the code easier to understand for other programmers.
Library Functions
In C programming, a library function is a set of pre-written functions that are included in the standard C libraries. These libraries are full with functions that can be used for a wide range of activities, from basic input/output operations to complex calculations and manipulating strings.
Overview of Standard C Libraries
The C programming language includes various standard libraries, each with a distinct function. Some of the often used standard libraries are:
- Stdio.h: This library contains routines for standard input and output operations. This library has functions like printf() and scanf().
- stdlib.h: This file provides functions for memory allocation, random number generation, and other utility functions like as atoi() and rand.
- Math.h: This library contains mathematical functions such as sin(), cos(), sqrt(), and others to perform mathematical computations.
- string.h: This library includes string manipulation functions such as strcpy(), strcat(), strlen(), and more.
- ctype.h: This file includes character handling routines such as isalpha(), isdigit(), and toupper().
How to Use Library Functions:
Using library functions in your C program involves two main steps:
- Including the Appropriate Header File: At the start of your programme, you must include the appropriate header file if you want to use any library functions. To use functions from the stdio.h library, use the line #include stdio.h> in your code.
- Calling the Functions: You can call the library functions directly from your programme after you’ve included the required header files. For example, you can use the printf() function from the stdio.h library to print output to the console.
Example: Using Library Functions:
#include <stdio.h> // Include the stdio.h header file
int main() {
int num;
printf("Enter a number: "); // Using printf() from stdio.h to display a message
scanf("%d", &num); // Using scanf() from stdio.h to read input from the user
printf("The square of %d is %d\n", num, num * num); // Performing calculations and displaying output
return 0;
}
Advantages of Library Functions:
- Time-saving: Library functions save time by offering pre-written code for often performed operations.
- Reliability: Standard library functions have been thoroughly tested and optimised for performance.
- Portability: Because standard libraries are included in the C language specification, code that uses them can be simply converted to various platforms.