Welcome to the wonderful world of PHP data types. Understanding data types is essential when start to learn programming. In this blog post, we’ll walk you through the various PHP data types, starting with simple integers and texts and on to complex arrays and objects. You will discover the significance of data types, how they affect your code, and how to use them efficiently in PHP projects. So join us as we learn the fundamentals of PHP data types, giving you the knowledge and confidence to face your coding issues head on. Now let’s get started!
Table of Contents
What is PHP data types?
The various values that can be saved and modified in PHP programming are referred to as PHP data types. These data types specify the nature of the data and how the PHP interpreter handles it. There are several types of data in PHP, each serving a specific purpose.
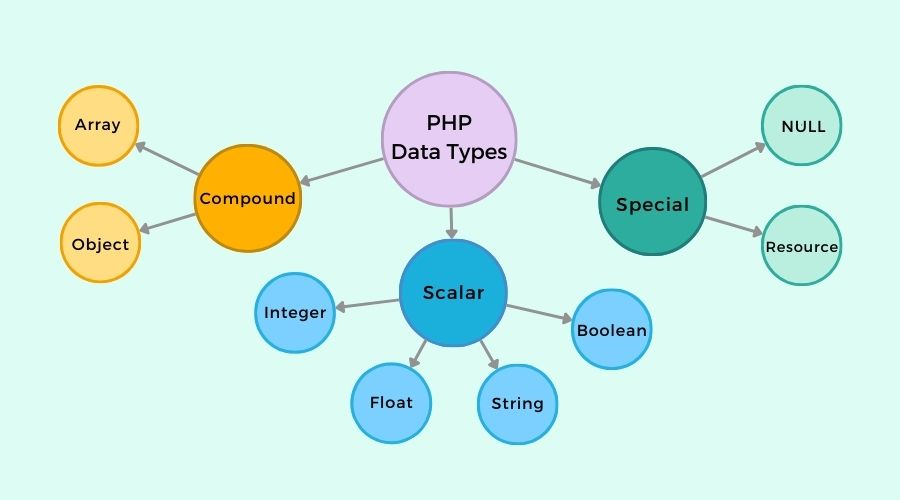
Scalar Data Types
Scalar data types in PHP store a single value at a time. These types serve as the foundation for PHP programming and include:
- Integer: A whole number with no decimal point. It can be positive, negative, or zero. For example, 5, -10, and 0 are all integers.
$num1 = 10; // Positive integer
$num2 = -5; // Negative integer
$num3 = 0; // Zero
- Float (Floating Point Number): Floats, also known as floating-point numbers or decimals, are numbers that have a fractional element. They are useful for representing numbers with decimals.
$float1 = 3.14; // Pi (approximation)
$float2 = -2.5; // Negative float
- String: A string is a series of characters, including numbers, letters, and symbols, that are encircled by double or single quotes (” ” or “‘”). Text data is represented as strings.
$name = "John"; // String with double quotes
$message = 'Hello, PHP'; // String with single quotes
- Boolean: A value that is either true or false is represented by a Boolean data type. Booleans are frequently used in logical processes and conditional statements.
$is_active = true; // Boolean true
$is_admin = false; // Boolean false
Compound Data Types
Compound data types in PHP, such as arrays and objects, enable you to store and organise multiple variables.
- Array: A collection of elements, each uniquely identified by an index, is called an array. An array can include a variety of data kinds, including numbers, strings, and other arrays. Here’s an example of how to create and use an array:
Example:
// Creating an array
$fruits = array("Apple", "Banana", "Orange");
// Accessing elements in the array
echo $fruits[0]; // Outputs: Apple
echo $fruits[1]; // Outputs: Banana
echo $fruits[2]; // Outputs: Orange
// Adding a new element to the array
$fruits[] = "Grapes";
echo $fruits[3]; // Outputs: Grapes
- Object: An object is a class instance that contains properties and methods. It enables you to design unique data structures and work with them as needed. Here is a simple example:
Example:
// Defining a class
class Person {
public $name;
public $age;
// Constructor function
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
// Method to display information
public function displayInfo() {
echo "Name: " . $this->name . ", Age: " . $this->age;
}
}
// Creating an object (instance) of the class
$person1 = new Person("John", 30);
// Accessing object properties
echo $person1->name; // Outputs: John
echo $person1->age; // Outputs: 30
// Calling object methods
$person1->displayInfo(); // Outputs: Name: John, Age: 30
Arrays and objects are crucial for managing complicated data structures in PHP, and knowing how to utilize them correctly is critical for developing dynamic and interactive online applications.
Special Data Types
- NULL: A unique data type in PHP called NULL represents a variable that has no value. It indicates that the variable has been intentionally set to NULL or has yet to be assigned a value.
Example:
$variable = NULL;
In this example, $variable
is explicitly set to NULL, indicating that it doesn’t contain any value.
- Resource: In PHP, an external resource, such as a file handle, database connection, or image canvas, is represented by a particular data type called a resource. Usually, external libraries or functions are responsible for creating and managing resources.
Example:
$file = fopen("example.txt", "r");
Here, $file
is a resource that represents the file “example.txt” opened for reading ("r"
) using the fopen()
function. This resource allows you to perform operations like reading from or writing to the file.
These special data types play crucial roles in PHP programming, especially when dealing with scenarios where variables may not have values or when interacting with external resources like files or databases.
Type Juggling and Type Casting in PHP
Type juggling and type casting are two PHP concepts that describe how PHP automatically changes data from one type to another.
Type Juggling:
Type juggling in PHP is the automatic conversion of data from one type to another as needed. For example, PHP will automatically convert a string to an integer and conduct the addition if you add a string and an integer together.
$number = 10;
$string = "20";
$result = $number + $string; // PHP automatically converts $string to an integer and adds it to $number
echo $result; // Output: 30
Type Casting:
Type casting, on the other hand, takes place when a programmer explicitly converts data from one type to another. This allows you to specify how data is transformed.
$number = 10;
$string = "20";
$intValue = (int) $string; // Type casting string to integer
$result = $number + $intValue;
echo $result; // Output: 30
In this example, (int)
is used to explicitly cast the string to an integer before performing the addition. Type casting gives you more control over the data conversion process compared to type juggling.
Also Read: Exploring the Depths of PHP Math Functions: A Beginner’s Guide
Determining Data Types
In PHP, determining the type of data you’re working with is critical for efficient programming. Gettype() and var_dump() are two typical functions used for this purpose.
1. gettype()
Function:
- The PHP method gettype() is used to find out a variable’s data type.
- It returns a string that contains the variable’s type, such as “integer,” “string,” “array,” etc.
$x = 10;
echo gettype($x); // Output: integer
2. var_dump()
Function:
- Var_dump() is another PHP function that returns detailed information about one or more variables, such as their data type and value.
- It provides structured information about the variable(s), such as the type, size, and value.
- This function is particularly useful for debugging and understanding the structure of complex data.
$x = 10;
$y = "Hello";
var_dump($x, $y);
/* Output:
int(10)
string(5) "Hello"
*/