Functions are important in the world of PHP programming. They are useful tools that allow us to complete specific tasks more efficiently. However, not every PHP function is the same. They are classified into many categories, each having a certain purpose and providing unique skills.
Any developer who wants to write clear, well-structured, and maintainable code must understand PHP function types. We’ll explore all of the various types of PHP functions in this tutorial, including user-defined functions created by programmers just like you and built-in functions included with the language.
We’ll go over the syntax, usage, and practical examples for each function type, making complex concepts easier to understand. By the end of this tutorial, you’ll have a thorough understanding of PHP function types, allowing you to use them more effectively in your projects. Now let’s get started!
Table of Contents
Basic Function Types in PHP
PHP functions are reusable code blocks that perform a specific task. They help to organise code, make it more readable, and reduce repetition. PHP has two main types of functions: user-defined functions and built-in functions.
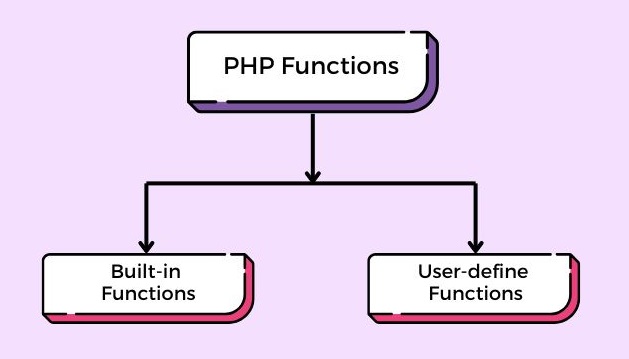
1. Built-in Functions:
Built-in functions are those that PHP already provides. These functions are ready to use and can handle a variety of tasks, including string manipulation, array work, date handling, and more. Built-in functions include date() for formatting dates, array_push() for adding entries to an array, and strlen() to calculate the length of a string.
PHP developers can use these functions instead of starting from scratch when writing code; they’re like tools in a toolbox. They are efficient and perform well, making them ideal for basic PHP programming jobs.
Exploring Commonly Used Built-in Functions
- String Functions
strlen()
: Returns the length of a string.strtolower()
,strtoupper()
: Converts a string to lowercase or uppercase.substr()
: Extracts a portion of a string.strpos()
,str_replace()
: Finds the position of the first occurrence of a substring or replaces occurrences of a substring.
- Array Functions
count()
: Returns the number of elements in an array.array_push()
,array_pop()
: Adds elements to the end of an array or removes the last element.array_merge()
,array_combine()
: Merges arrays or combines two arrays into one.array_key_exists()
: Checks if a specified key exists in an array.
- Math Functions
abs()
: Returns the absolute value of a number.round()
,ceil()
,floor()
: Rounds a floating-point number to the nearest integer, up, or down.rand()
: Generates a random integer.sqrt()
,pow()
: Calculates the square root or raises a number to a power.
- Date and Time Functions
date()
: Formats a timestamp into a human-readable date string.time()
,strtotime()
: Returns the current Unix timestamp or converts a date string into a timestamp.strtotime()
: Converts a human-readable date/time string into a Unix timestamp.
- File System Functions
file_exists()
: Checks if a file or directory exists.file_get_contents()
,file_put_contents()
: Reads from or writes data to a file.unlink()
: Deletes a file.
- Other Useful Functions
isset()
,empty()
: Checks if a variable is set and not empty.htmlspecialchars()
,urlencode()
: Converts special characters to HTML entities or encodes a URL.
2. User-defined Functions:
User-defined functions are ones that the developer creates based on their own requirements. These features enable programmers to create custom instructions for carrying out specific tasks.
User-defined functions add flexibility and modularity to the code, allowing developers to divide difficult tasks into smaller, more manageable portions. They encourage code reuse, readability, and maintainability, making the codebase more understandable and maintainable over time.
Syntax for Defining User-defined Functions:
function functionName(parameter1, parameter2, ...) {
// Function body - code to be executed
// Optionally, return a value
}
- functionName: This is the function’s name. Select a name for the function that accurately describes its functions.
- parameters: These are optional. You can provide values into a function using parameters. You can have zero, one, or more parameters separated by commas.
- Function Body: This is where you write the code that will run when the function is called.
- Return Statement (Optional): You can optionally include a return statement in your function to return a value to the caller code.
Parameters and Return Values in User-defined Functions:
Parameters allow functions to accept input values that can be used within the function. Return values allow functions to return results after processing.
- Parameters:
- Parameters are variables that store the values provided to a function when it is called.
- These values can be used in the function to perform operations.
- In a function definition, parameters are enclosed in parenthesis.
- They serve as placeholders for the values to be used in the function.
Example:
function greet($name) {
echo "Hello, $name!";
}
// Calling the function
greet("John"); // Output: Hello, John!
- Return Values:
- Within a function, data can be sent back to the calling code via return values.
- They help functions to execute tasks and provide results to the code that called them.
- Return values are defined by using the return keyword followed by the value to be returned.
- When a return statement is found, the function execution is terminated and control returns to the calling code.
Example:
function add($num1, $num2) {
$sum = $num1 + $num2;
return $sum;
}
// Calling the function and storing the result
$result = add(5, 3);
echo $result; // Output: 8
Built-in Functions vs. User-defined Functions
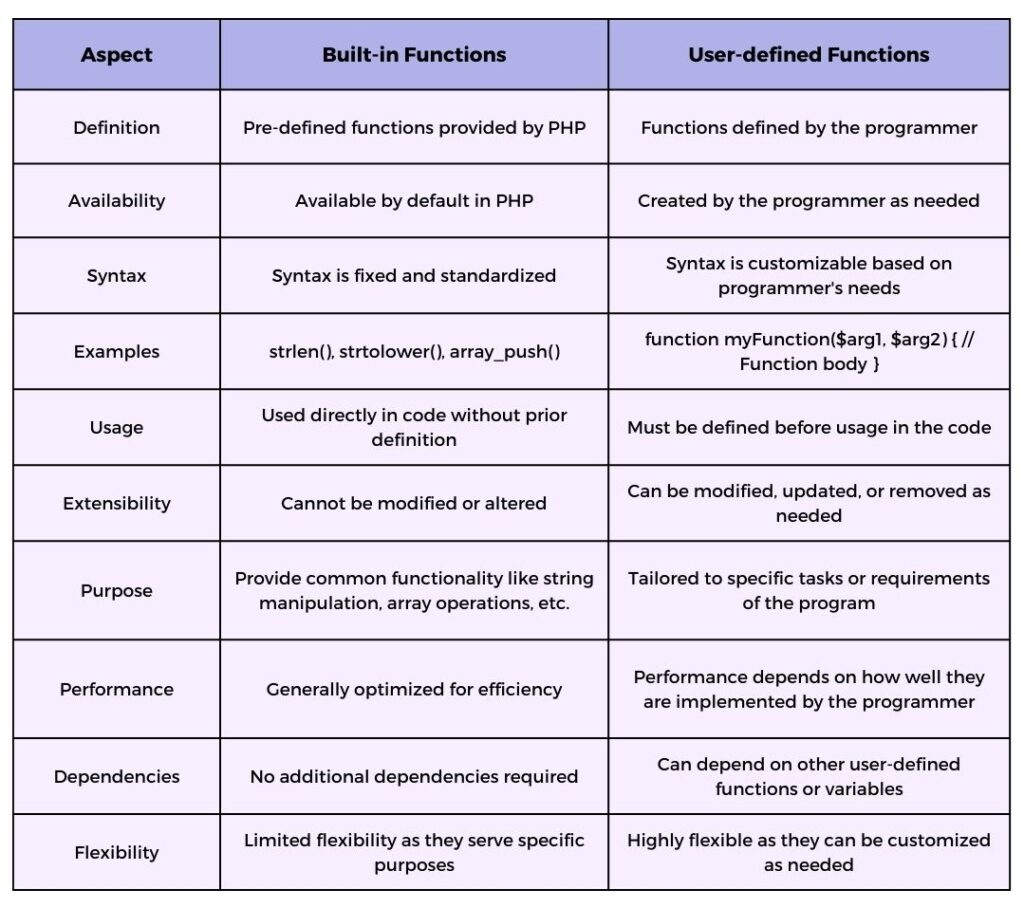
Advanced Function Types
1. Recursive Functions:
Recursive functions call themselves within their own definition. They’re especially beneficial for resolving problems that can be divided into smaller, related subproblems. Here’s a simple explanation.
- Imagine you have a task that can be broken down into smaller tasks of the same type.
- You solve each smaller task separately until you reach the simplest example (the basic case).
- Then you add the results of all the smaller tasks to solve the original problem.
Example: Calculating factorial.
function factorial($n) {
if ($n <= 1) {
return 1; // Base case: factorial of 0 or 1 is 1
} else {
return $n * factorial($n - 1); // Recursive call
}
}
2. Anonymous Functions (Closures):
Anonymous functions, often known as closures, are functions with no name. They are frequently employed in situations when a callback function is necessary or when one function must be sent as an input to another. This is a simplified explanation:
- Anonymous functions allow you to define a function on the fly without assigning it a name.
- They can capture variables from the surrounding scope, making them ideal for developing flexible and reusable programmes.
Example: Sorting an array using usort()
with an anonymous function.
$numbers = [3, 1, 2, 5, 4];
usort($numbers, function($a, $b) {
return $a - $b;
});
print_r($numbers); // Output: [1, 2, 3, 4, 5]
In this example, the anonymous function inside usort()
defines the custom sorting logic without needing a separate named function.