In programming, data types are the foundation for storing and manipulating information. Computers organize data into different types to conduct various operations efficiently, just as people do in real life by classifying objects into categories such as numbers, letters, or words. In C programming, data types specify the sort of data that a variable can store and the actions that can be performed on it. Understanding data types is essential for designing efficient and error-free programs.
We will go into the basics of data types in this section, explaining what they are, why they are important, and how they shape the way we write code. This overview will provide you with a strong basis for understanding data types in C, whatever your level of experience with coding. Now let’s get started!
Table of Contents
what is Data Type in c
In C programming, a data type is similar to a label that tells the compiler what kind of data a variable contains. It allows the compiler to allocate memory and understand how to interpret data. For example, int is a data type that stores whole numbers, float stores decimal values, and char stores single characters such as letters or symbols. Data types help to verify that variable operations are performed correctly, reducing errors and making code easier to understand. Correct data types help in managing memory efficiently, performing operations accurately, and preventing errors.
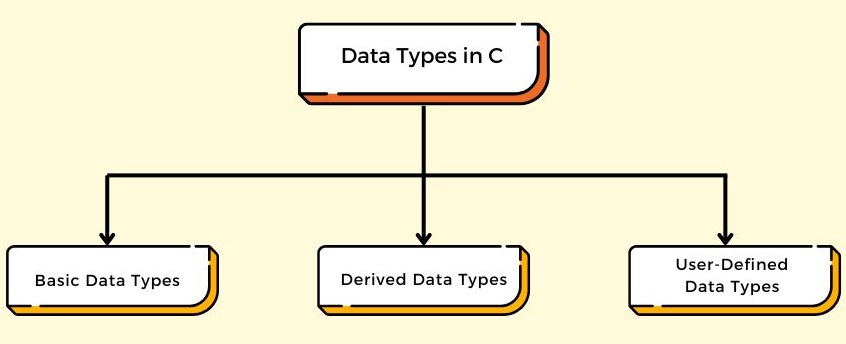
Basic Data Types
1. Integers:
Integers are whole numbers with no decimal points. In C, you can define integers with the int keyword. For example:
int age = 25;
Here, age
is an integer variable with the value 25.
2. Floats:
Decimal points can be represented using floating-point numbers, or floats. You can use the float keyword in C to define floats. For example:
float price = 10.99;
Here, price
is a float variable with the value 10.99.
3. Characters:
Characters are individual representations of letters, numbers, or symbols. With the char keyword in C, you can define characters. For example:
char grade = 'A';
Here, grade
is a character variable with the value ‘A’.
These data types are key building blocks for C programming and are widely used in a variety of applications. Integers are used for counting and indexing, floats for representing fractional numbers, and characters for textual data.
Derived Data Types
In C, derived data types such as arrays and pointers are essential for efficiently managing data collections.
1. Arrays:
Arrays are collections of elements with the same data type that are stored in contiguous memory locations. They make it possible to store several values under a single name. For Example:
int numbers[5]; // Declares an array 'numbers' capable of storing 5 integers
numbers[0] = 10; // Assigns 10 to the first element of the array
numbers[1] = 20; // Assigns 20 to the second element of the array
// ...
2. Pointers:
Pointers are variables that contain memory addresses. They are used to store addresses of other variables or functions, allowing for more flexibility and efficiency in memory management.
For example:
int *ptr; // Declares a pointer variable 'ptr' capable of storing the address of an integer
int num = 100;
ptr = # // Stores the address of 'num' in the pointer variable 'ptr'
printf("Value of num: %d\n", num); // Prints the value of 'num'
printf("Address of num: %p\n", &num); // Prints the address of 'num'
printf("Value pointed by ptr: %d\n", *ptr); // Prints the value pointed to by 'ptr'
Understanding arrays and pointers is essential for effective memory management and manipulation of data structures in C programming.
User-Defined Data Types
Programmers can design unique data structures and enumerations using C’s User-Defined Data Types, which improve readability and code organisation.
1. Structs:
A struct is a composite data type that combines variables from different data types under a single name. Consider it a container for linked data fields.
For Example:
// Defining a struct to represent a person
struct Person {
char name[50];
int age;
float height;
};
The struct Person, which has three members—name, age, and height—is defined in this example. Each member has its own data type.
2. Enums:
Enums (short for enumerations) allow you to define a set of named integer constants. They improve code readability and maintainability by assigning meaningful names to integral values.
Example:
// Defining an enum for days of the week
enum Days {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
};
In this example, we’ve created an enum called Days with seven constants that represent the days of the week. By default, the first constant (MONDAY) is set to 0, the second (TUESDAY) is set to 1, and so on.
Usage:
// Example usage of structs and enums
#include <stdio.h>
int main() {
// Declaring a struct variable
struct Person person1;
// Assigning values to struct members
strcpy(person1.name, "John");
person1.age = 30;
person1.height = 6.2;
// Printing struct values
printf("Name: %s\n", person1.name);
printf("Age: %d\n", person1.age);
printf("Height: %.1f\n", person1.height);
// Declaring an enum variable
enum Days today;
// Assigning a value to the enum variable
today = WEDNESDAY;
// Printing the value of the enum variable
printf("Today is: %d\n", today);
return 0;
}
In this usage example, we declare a struct variable person1
of type Person
, assign values to its members, and print them. Then, we declare an enum variable today
, assign the value WEDNESDAY
to it, and print its value.
By using structs and enums, programmers can create more organized and expressive code structures in C.
Size and Range of Data Types
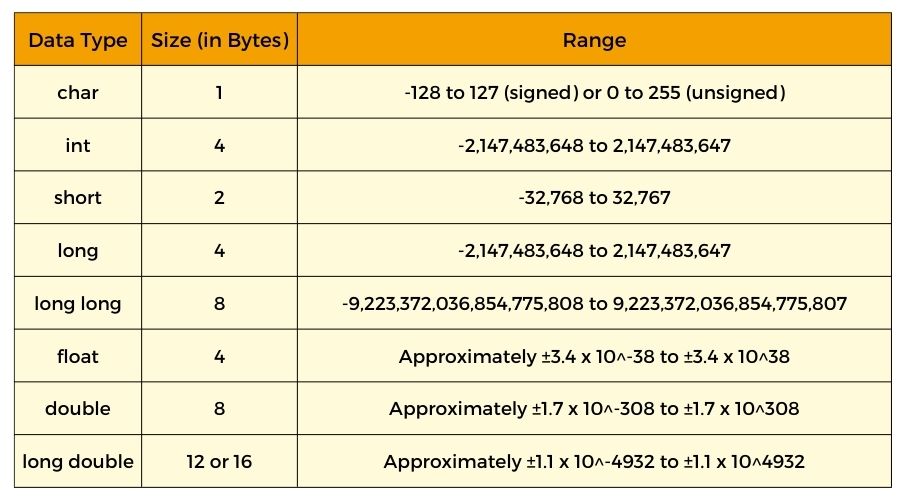
Type Casting: Converting Data Types
In programming, type casting is the conversion of one data type to another. This is useful when you need to do operations or comparisons with various sorts of data. Here’s a basic explanation with an example:
Assume you have two variables: num1, which stores an integer (whole number), and num2, which stores a floating-point (decimal).
int num1 = 10;
float num2 = 3.5;
Now, suppose you wish to conduct a calculation that requires num1 to be handled as a floating-point number. You can accomplish this by converting num1 to a float before performing the calculation.
float result = (float)num1 * num2;
In this instance, the type cast is (float)num1. It tells the compiler not to consider num1 as an integer, but as a float. Therefore, floating-point arithmetic is used to complete the multiplication operation, and the result is saved in the result variable.
Similarly, you can cast variables to various data types such as integers, characters, and so on based on your needs. Just make sure to use the appropriate cast syntax (desired_type) variable_name.