Unity 3D, a popular game engine, offers a powerful tool called coroutines that allow you to create asynchronous tasks without blocking the main thread, which is essential for maintaining smooth gameplay and responsiveness. Unity 3D, a popular game engine, offers a powerful tool called coroutines that allow you to create asynchronous tasks without blocking the main thread, which is essential for maintaining smooth gameplay and responsiveness.This can be extremely useful for handling asynchronous tasks, animations, delays, and other situations where you need to pause and resume code execution without freezing the entire application. In this beginner-friendly guide, we will dive into the world of coroutines, exploring how they can enhance your Unity 3D development experience.
Table of Contents
Introduction to Coroutines
Coroutines are a unique feature in Unity’s scripting environment that provide a structured way to perform asynchronous tasks while still maintaining control over the execution flow.
Unlike traditional functions, which run to completion in one frame, coroutines allow you to pause their execution, yield control back to the game loop, and then resume from where they left off. This enables you to create complex, time-based behaviours without resorting to complex threading or freezing the main thread.
In Unity 3D, a coroutine is a special type of function that returns an IEnumerator
. The yield
keyword is used within coroutines to signal when to pause execution and when to resume. This enables you to break down complex operations into smaller, manageable steps and perform them over multiple frames.
Key Characteristics of Coroutines
1. Non-Blocking: Coroutines execute in a way that doesn’t block the main thread, which is crucial for maintaining smooth gameplay and responsive user interactions.
2. Frame-Based Execution: Coroutines run in sync with the game’s frame rate. You can use yield return null;
to pause and resume the coroutine on each frame, enabling you to spread out work across multiple frames
3. Yield Instructions: The yield
keyword is used with different instructions like WaitForSeconds
, WaitForFixedUpdate
, and WaitForEndOfFrame
to control the timing of pauses and resumes.
4.Time-Management: Coroutines are excellent for handling timed actions, animations, delays, and transitions. They allow you to control when things happen in your game with precision.
5. Main Thread Safety: Unity’s coroutines ensure that any code executed within them is performed on the main thread. This is crucial for avoiding threading-related issues.
Getting Started: Your First Coroutine
This section will guide you through setting up your development environment, creating a straightforward coroutine, and running it inside of Unity.
1.Setting up your development environment
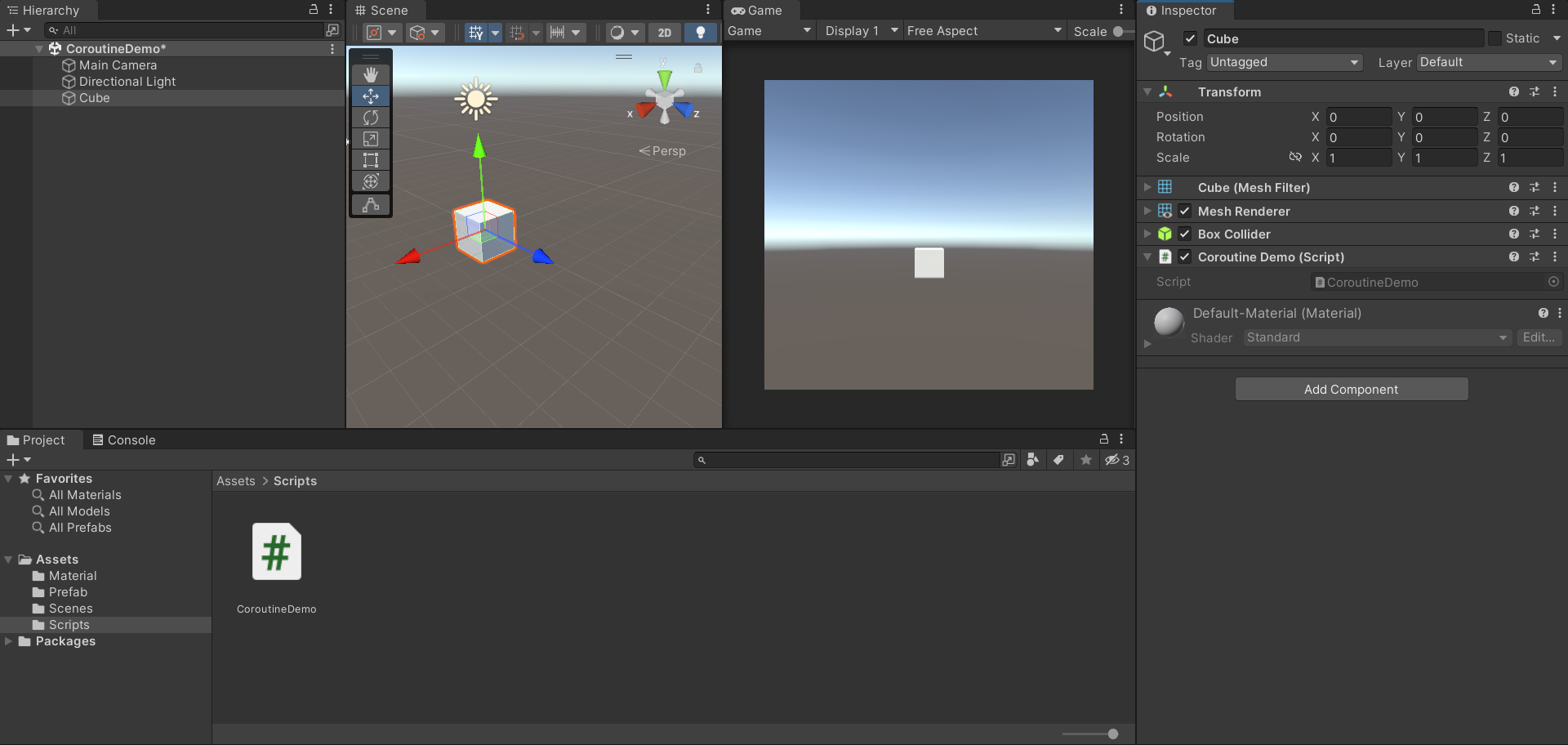
1. Open Unity : Launch Unity and open your project, or create a new one by clicking “New” in the Unity Hub.
2. Create or Select an Object: Select an object in the Hierarchy panel with which you want to associate the coroutine. This object could be anything you wish to manipulate or animate over time.
3. Create a C# Script: Right-click in the Assets panel, choose “Create,” and then select “C# Script. “Give the script a name like “CoroutineDemo.”
4. Attach the Script: To open the script in your preferred code editor, double-click it. Alternatively, you can drag and drop the script onto the object you selected in the Hierarchy panel to attach it.
2. Writing a simple coroutine
Now, let’s write a basic coroutine that demonstrates the concept:
using UnityEngine;
using System.Collections;
public class CoroutineDemo : MonoBehaviour
{
private IEnumerator MyFirstCoroutine()
{
Debug.Log("Coroutine started!");
yield return new WaitForSeconds(2f); // Pause for 2 seconds
Debug.Log("Coroutine resumed after waiting!");
// Additional coroutine logic can go here
}
}
In this script, we’ve defined a coroutine named MyFirstCoroutine. It logs a message, pauses for 2 seconds using yield return new WaitForSeconds(2f);, and then logs another message. You can customize this coroutine to include the behavior you want.
3. Running coroutines in Unity
A coroutine must be called from another function, usually the Start method, in order to be executed. Let’s modify our script to run the coroutine:
using UnityEngine;
using System.Collections;
public class CoroutineDemo : MonoBehaviour
{
private IEnumerator MyFirstCoroutine()
{
Debug.Log("Coroutine started!");
yield return new WaitForSeconds(2f);
Debug.Log("Coroutine resumed after waiting!");
// Additional coroutine logic can go here
}
private void Start()
{
StartCoroutine(MyFirstCoroutine()); // Start the coroutine
}
}
In this updated script, we’ve added a Start method. Inside Start, we call the coroutine using StartCoroutine(MyFirstCoroutine());.
4. Testing the coroutine
- Save and Compile : Save your script, then come back to Unity. The script is recompiled by Unity automatically.
- Play the Scene: To run your scene, click the “Play” button at the top of the Unity window.
- Observe the Console: You’ll see the debug messages from your coroutine displaying in the Console panel. Before resuming and showing the second message, the coroutine will pause for two seconds.

You’ve successfully written and run your first Unity 3D coroutine! This simple example shows how to set up your environment, create a time-delayed coroutine, and start it inside the Start method. You are now prepared to explore more complex applications of coroutines, such as managing animations, timed actions, and other asynchronous processes to improve your Unity 3D projects.
Yield Instructions
The power of coroutines comes from their ability to yield instructions. You can use various yield instructions to specify when and how the coroutine should yield control back to the engine. Some common yield instructions include:
yield return null;
: Yields control until the next frame.yield return new WaitForSeconds(seconds);
: Yields control for a specified time.yield return new WaitForEndOfFrame();
: Yields control after all rendering is done in the current frame.yield return new WaitForFixedUpdate();
: Yields control at the next FixedUpdate call (useful for physics calculations).
Coroutines empower Unity beginners to tackle complex tasks and enhance their games’ responsiveness and interactivity. Let’s use the useful magic of coroutines to unlock the full power of your Unity projects!