If you’re new to coding or want to improve your programming skills, understanding data types is the first and most important step. Data types are like the keys to a puzzle in the world of C programming; they open the door to the possibility of writing strong and effective code. Think of them as the various tools in your programming toolbox, each with a specific function. Our goal in this blog article is to demystify C datatypes by breaking down their complexity and explaining how they are the foundation of efficient programming.
A strong understanding of datatypes is essential whether you’re a new coder or an experienced developer. It’s the language that your computer speaks, and developing clear, efficient, and error-free code starts with becoming proficient in it. So buckle up as we explore the complexities of C datatypes and open the path for you to develop a solid programming foundation. Now let’s get started!
Table of Contents
Importance of understanding Data types in C
Datatypes are an essential cornerstone in the broad world of C programming, where complex algorithms and functionality are linked together by lines of code. Consider datatypes as the fundamental units of your program, each with a distinct function and size that enhances its overall structure and performance.
Why Do Data types Matter?
In C, datatypes are similar to toolkit containers. They specify the types of data that can be stored in variables, including decimals, characters, and whole numbers. Because these datatypes add accuracy and efficiency to your code, it is important to understand them.
Consider this: if your program is a language, datatypes are its grammar. Just as proper grammar ensures clear communication, selecting the appropriate datatype ensures that your program communicates effectively with the computer.
Let’s now imagine your code as a house. The datatype is the blueprint that determines how your house is built, not just the material you choose. If you select the incorrect datatype, your program won’t be able to withstand the demands of the real world. It would be like trying to build a skyscraper with toothpicks.
What are Data types in C?
Datatypes are the foundation for organizing and manipulating data in the field of C programming. In essence, a datatype specifies the kinds of values that can be stored in a variable and the actions that can be performed with those values.
In simplest terms, datatypes are a collection of rules that define how a computer interprets and interacts with various types of data. For example, a character datatype works with letters and symbols, whereas an integer datatype deals with numbers.
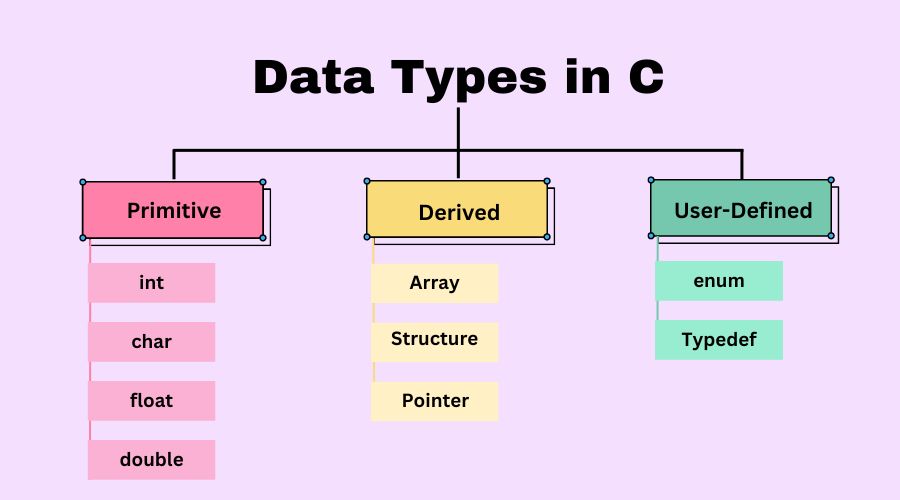
In C programming, datatypes can be broadly classified into three main categories:
- Primitive Data types:
- int: Used for whole numbers.
- char: Manages a single character.
- float: Handles decimal point-containing numbers.
- double: Like float, but with more precision.
- Derived Data types:
- Arrays: Sequentially organizes multiple data of the same type.
- Structures: Organize several datatypes under a single heading.
- Pointers: Increase the flexibility of manipulating variables by storing memory addresses.
- User-Defined Data types:
- Enumerations (enum): Defines a set of named integer constants to improve code readability.
- Typedefs: Allows you to create custom names for existing datatypes, which improves code readability and maintainability.
Basic Data types in C
Datatypes in C programming define the type of data a variable can hold , and each datatype consumes a particular amount of memory. It is essential to understand datatype sizes in order to use memory effectively.
Datatype | Size (in bytes) | Range | Format Specifier |
---|---|---|---|
int | 4 | -2,147,483,648 to 2,147,483,647 | %d |
char | 1 | 0 to 255 (or -128 to 127) | %c |
float | 4 | 3.4e-38 to 3.4e+38 | %f |
double | 8 | 1.7e-308 to 1.7e+308 | %lf |
short int | 2 | -32,768 to 32,767 | %hd |
long int | 4 or 8 | -2,147,483,648 to 2,147,483,647 | %ld |
long long int | 8 | -(2^63) to (2^63)-1 | %lld |
Explanation:
- Size (in bytes): The amount of memory occupied by each datatype..
- Range: The set of values that a variable of that datatype can hold.
- Format Specifier: The placeholder that the printf() and scanf() functions use to indicate the kind of data.
The range for char can be defined as either -128 to 127 for a signed char or 0 to 255 for an unsigned char.
For floating-point types (float and double), the range shows the magnitude rather than the accuracy.
Datatype sizes may change between systems and compilers.
Sure, let’s delve into each basic datatype in C with simple explanations and examples:
1. Integer Data type (int
):
The Integer datatype is used to store whole numbers without decimal points.
Example: If you’re counting the number of students in a class, you might use an int
variable.
#include <stdio.h>
int main() {
int numberOfStudents = 30; // Declaration and initialization of an int variable
printf("Number of students: %d\n", numberOfStudents); // Printing the value
return 0;
}
2. Character Data type (char
):
The Character
datatype is used to store single characters, like letters or symbols.
Example: Storing a grade or representing a single character.
#include <stdio.h>
int main() {
char grade = 'A'; // Declaration and initialization of a char variable
printf("Grade: %c\n", grade); // Printing the value
return 0;
}
3. Floating-point Data type (float
):
The Float
datatype is used to store numbers with decimal points.
Example: If you’re dealing with a value like 3.14 for calculations, you might use a float
variable.
#include <stdio.h>
int main() {
float piValue = 3.14; // Declaration and initialization of a float variable
printf("Value of pi: %f\n", piValue); // Printing the value
return 0;
}
4. Double Precision Floating-point Data type (double
):
The double
datatype is similar to float
but offers more precision for decimal values.
Example: When high precision is required, like in scientific calculations, you might use a double
variable.
#include <stdio.h>
int main() {
double largeNumber = 123456789.987654321; // Declaration and initialization of a double variable
printf("Large number: %lf\n", largeNumber); // Printing the value
return 0;
}
Derived Data types
Derived datatypes are constructs that build on primitive datatypes, allowing programmers to create more complex and structured variables to meet specific needs.
1. Arrays:
Arrays are collections of elements of the same datatype that may be accessed using an index or a key. In C, arrays are essential derived datatypes that let you store multiple elements of the same type together under a single name. They offer an organized method of organizing and accessing data.
Example:
int numbers[5] = {1, 2, 3, 4, 5};
This declares an integer array named numbers
with five elements.
Arrays are useful for dealing with data sets such as lists or matrices. An array’s elements are accessible by an index, which starts at 0. Arrays improve the structure and efficiency of programming.
2. Structures:
Structures allow grouping different datatypes under a single name. A composite datatype, or structure, unites variables of many kinds under a common name. Every variable in the structure is called a member.
Example:
struct Student {
char name[50];
int age;
float GPA;
};
This defines a structure named Student
with members name
, age
, and gpa
.
Structures enable the grouping of several forms of data into a single structure. They make it easier to create more complex data entities, such as people having names, ages, and heights. A member is an element of data within a structure. Members of a structure can have different data types. The dot notation is used to access structural members (student1.age).
3. Pointers:
In C, pointers are strong derived datatypes used to hold addresses in memory. They make it possible to allocate and manipulate memory dynamically. A variable that holds the memory address of another variable is called a pointer. It allows you to access a value stored in memory indirectly.
Example:
int num = 10;
int *ptr = #
Here, ptr
is a pointer that stores the address of num
.
Pointers provide immediate access to and manipulation of data by storing memory addresses.
They are essential for effective data processing and dynamic memory allocation. Pointers improve the efficiency and flexibility of C programs. To find a variable’s address, use the address-of operator (&).
To access the value stored at a pointer, use the value-at-address operator (*).
User-Defined Data types
User-defined datatypes in C allow programmers to construct new data types that are suited to their individual requirements, improving code readability and maintainability.
1. Enums (Enumerations):
A user-defined data type made up of named integral constants is called an enum. They enable the creation of symbolic names for collections of integer values.
Example:
enum Weekdays {
Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday
};
Here, Weekdays
is an enum type, and each day is assigned a unique integer value starting from 0. This improves code readability as you can use Monday
, Tuesday
, etc., instead of remembering numeric values.
2. Typedef:
Typedef allows programmers to define aliases for existing data types, making the code more self-explanatory and flexible.
typedef struct {
int day;
int month;
int year;
} Date;
In this example, typedef
creates an alias Date
for the structure containing day, month, and year. Now, instead of declaring variables like struct Date myDate
, you can simply use Date myDate
.
Enums and typedefs make code more human-readable by replacing numeric values with meaningful names. Typedefs make it easier to adapt code in the future. If the underlying data type needs to change, you only need to update the typedef.
Void Data type:
The void datatype is a unique and fascinating idea in C programming. Unlike other datatypes that carry values, void is unique in that it represents the absence of a specific type. In essence, the word “void” refers to nothing or empty space. Similar to this, the C language uses the symbol void to indicate that a function or reference has no associated type or does not return any value at all.
A function in C that is declared with the void keyword indicates that it does not return any values. As an example:
void sayHello() {
printf("Hello, World!\n");
}
Here, sayHello
is a function that doesn’t return anything. It’s just there to perform a set of tasks.
Data type Conversion
The process of transforming a variable’s datatype from one type to another is called datatype conversion, sometimes referred to as type casting. This is necessary when working with variables of multiple datatypes in C programming.
Types of Data type Conversion:
1. Implicit Conversion:
Implicit conversion, commonly referred to as “coercion,” occurs automatically when different datatypes are used in expressions. The conversion is handled by the compiler to ensure compatibility.
Example:
int integerNumber = 5;
float floatNumber = 2.5;
// Implicit conversion: int is automatically converted to float
float result = integerNumber + floatNumber;
In this example, the integerNumber
is implicitly converted to a float
before the addition operation.
2. Explicit Conversion (Casting):
Programmers specify the conversion from one datatype to another manually in an explicit conversion process, often known as casting. To ensure accuracy and control over the type conversion, casting operators are used in this process.
Example:
float floatNumber = 2.5;
int integerNumber;
// Explicit conversion: float is explicitly cast to int
integerNumber = (int)floatNumber;
Here, the programmer explicitly casts the floatNumber
to an int
using (int)
to truncate the decimal part and assign the result to integerNumber
.
In conclusion, developing effective and error-free programs requires an in-depth knowledge of C data types. Developers can optimize code and improve program speed by having a solid understanding of primitive and derived datatypes as well as memory allocation and conversion procedures. Programmers can create a solid foundation for their projects by following best practices, such as choosing the appropriate datatype for a given task and maintaining uniformity in coding standards.