Welcome to our comprehensive guide to C language keywords and their applications! If you’re new to C programming or want to improve your knowledge, you’ve come to the perfect place. Keywords are the foundation of any programming language, acting as essential building blocks for developing powerful and efficient code.
In this book, we’ll go over each essential keyword in C, describing what it does and how it’s utilized in real-world programming places. Whether you’re struggling with fundamental principles or looking for clarification on advanced topics, our goal is to demystify the world of C keywords through straightforward explanations and real-world examples.
By the end of this guide, you’ll have a basic understanding of the C language’s essential components, allowing you to develop cleaner, more efficient code and confidently tackle a variety of programming issues. Together, let’s go into and discover the exciting world of C language keywords!
Table of Contents
What are Keywords in C Language?
Within the C programming language, keywords—also referred to as reserved words—are predefined identifiers with specific meanings. These words are reserved by the language and cannot be used for anything else, such as naming variables or functions. Instead, they play specialized functions in determining the program’s structure, control flow, and functionality.
In C, some frequently used keywords are “int,” “char,” “if,” “else,” “for,” and “while.” Each keyword serves a specific purpose, such as declaring variables, managing programme flow with conditionals, or iterating through loops.
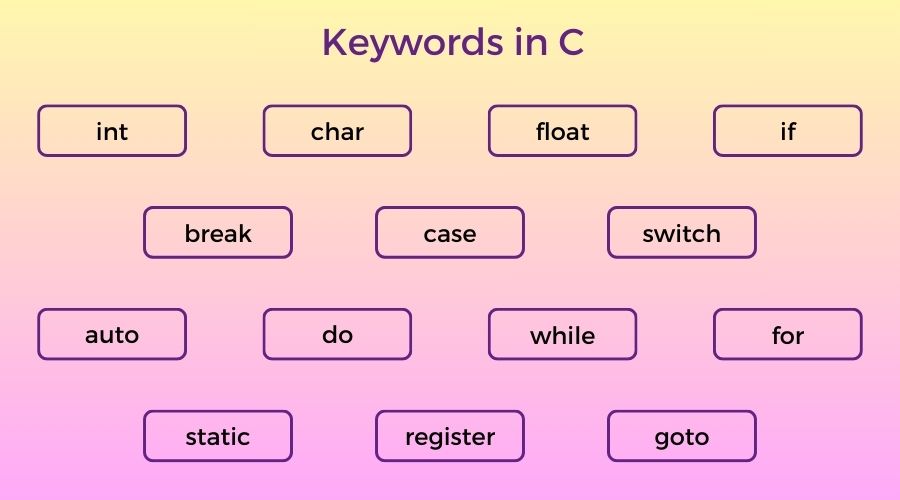
Importance of Understanding Keywords
Understanding the significance of keywords is essential for beginners to start with C programming. These reserved words offer the fundamental building blocks required to create logical and useful code. Understanding the roles and meanings of keywords helps to:
- Create valid and syntactically correct programmes.
- Use loops and control structures to effectively manage programme flow.
- Use the right data types when declaring and modifying variables.
- Understand and change existing codebases with confidence.
- Communicate efficiently with other programmers by following standard C syntax and practices.
In essence, learning keywords in C language allows beginners to communicate their programming ideas effectively and efficiently. It lays the framework for further research and expertise in C programming, allowing developers to confidently tackle increasingly difficult tasks.
Definition and Role of Keywords in Programming
Programming language keywords are special terms with specified meanings that are used only for specified purposes within the language. These terms cannot be used for any other reason, such as naming variables or functions, because they are a part of the language syntax. They act as building blocks for programmes and provide instructions to the compiler or interpreter on how to interpret and execute the code.
Keywords in C, such as “int”, “char”, “if”, “else”, “for”, and so on, have specified meanings. Because of these keywords’ specific function in the language, the compiler knows exactly what to do when you use them in your code.
How Keywords Differ from Identifiers and Variables
Identifiers and variables, on the other hand, are user-defined names assigned to entities such as variables, functions, or other programming elements in the code. Unlike keywords, identifiers can be chosen freely by the programmer as long as they follow to the rules and conventions established by the programming language.
Variables are data storage places used during programme execution. They can represent several kinds of data, including characters, integers, floating-point numbers, etc., and are named using identifiers.
In conclusion, identifiers and variables are user-defined names used to represent entities and hold data values within a program, whereas keywords have predetermined meanings and are reserved for certain tasks within the language syntax.
Commonly Used Keywords in C Programming
When programming in C, keywords are essential for defining the structure and behaviour of your code. Let’s look at some of the most often used keywords and what they do.
1. Basic Keywords:
- int: Used to specify integer variables. For example, int age = 25;.
- Char: Character variable declaration. For example, char grade = ‘A’.
- float: Used to define floating-point variables. For example, float price = 10.99.
2. Control Flow Keywords:
- if, else: Used for conditional execution. For example:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
- switch: Used for multi-way branching. For example:
switch (expression) {
case constant1:
// code to execute if expression equals constant1
break;
case constant2:
// code to execute if expression equals constant2
break;
default:
// code to execute if expression doesn't match any case
}
3. Iteration Keywords:
- for: Used for loop execution with a predetermined number of iterations. For example:
for (initialization; condition; increment/decrement) {
// code to execute repeatedly until the condition becomes false
}
- while: Used for loop execution with a condition. For example:
while (condition) {
// code to execute repeatedly until the condition becomes false
}
- do-while: Similar to the while loop, but guarantees that the loop body is executed at least once before checking the condition. For example:
do {
// code to execute repeatedly until the condition becomes false
} while (condition);
4. Storage Class Keywords:
- auto: Allocates memory for variables within a block.
- static: A variable’s value is kept constant between function calls.
- extern: Declares a variable defined in another file.
- register: Informs the compiler to save the variable in a CPU register for faster access.
5. Other Essential Keywords:
- return: Used to exit a function and return its value.
- Break: Used to terminate a loop or switch statement prematurely.
- Continue: Skips the rest of the loop’s body and moves on to the next iteration.
Also Read: Demystifying Functions in C Programming: A Comprehensive Guide
Syntax Rules for Using Keywords in C
- Reserved Words: The C language defines and reserves some keywords. They are not suitable for usage as variable names or identifiers.
- Case Sensitivity: C keywords are case-sensitive. For example, “int” is a keyword, but “INT” and “Int” are not.
- No Spaces: Keywords cannot contain spaces.
- Placement: Keywords should be used according with the program’s syntactic role. For example, “if” should be followed by a conditional statement enclosed in brackets.