For loops are a key idea in C programming that allows you to execute a block of code repeatedly. They are extremely strong tools for automating operations, iterating over data structures such as arrays, and addressing a variety of programming difficulties. In this blog post, we’ll look at for loops in C, including their syntax, uses, and how they can help you improve your coding skills. Whether you’re just beginning learning the fundamentals or an experienced programmer searching for advanced techniques, this article will help you master the art of for loops in C programming. Let’s get started.
Table of Contents
Basic Syntax and Structure of For Loops
In C programming, a for loop is used to execute a block of code repeatedly until a specific condition is met. A for loop’s syntax is divided into three main parts:
Initialization: This section sets a variable before the loop begins. It typically involves declaring a loop control variable and giving it an initial value.
Condition: This section indicates the condition that must be true for the loop to continue executing. If the condition returns false, the loop ends.
Increment/Decrement: This section modifies the loop control variable after each iteration of the loop. It has the ability to both increase and reduce the variable’s value.
Here’s the general structure of a for loop in C:
for (initialization; condition; increment/decrement) {
// Code to be executed repeatedly
}
Flow Chart of For Loop
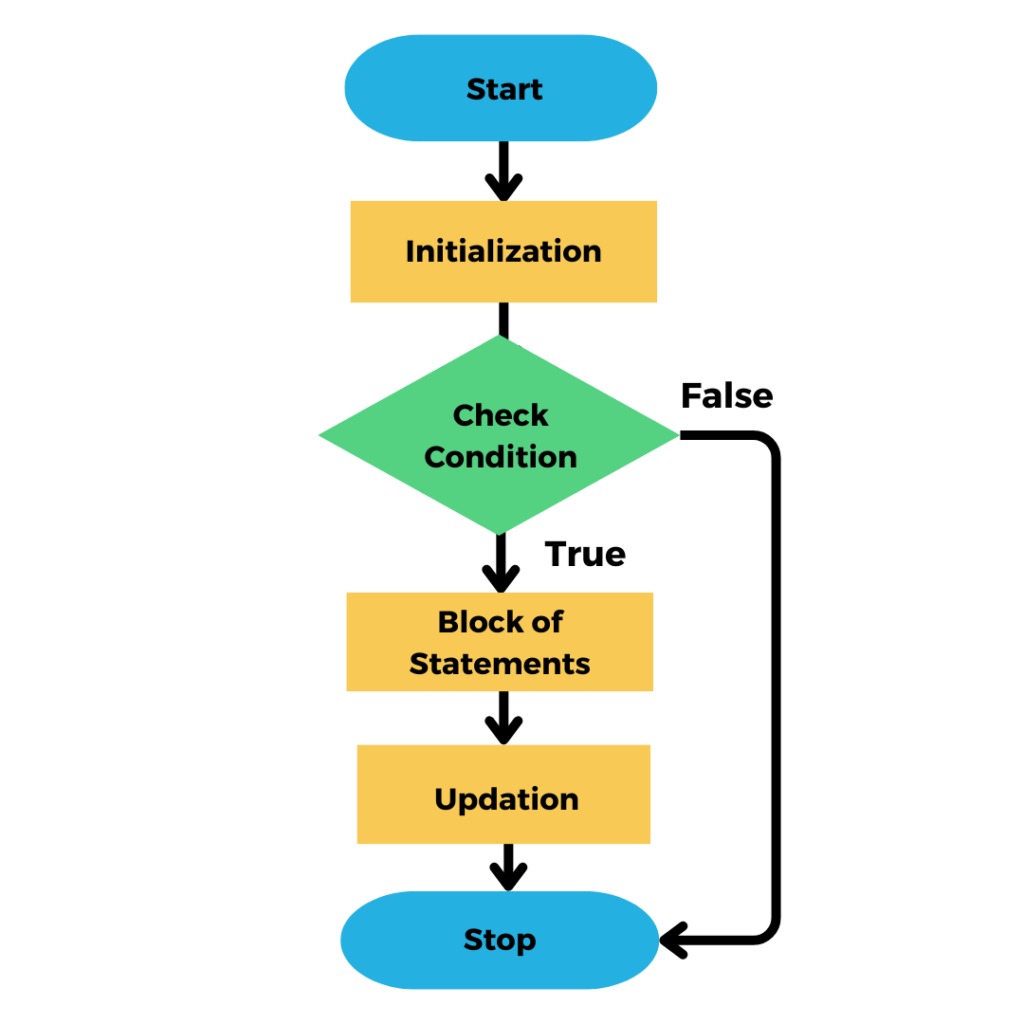
Example 1: Simple For Loop Implementation
For example, if you want to print numbers from 1 to 5
#include <stdio.h>
int main() {
int i; // Initialization
for (i = 1; i <= 5; i++) { // Condition; Increment
printf("%d\n", i); // Loop body: Print the current value of i
}
return 0;
}
This loop will run as long as i
is less than or equal to 5. In each iteration, i
is incremented by 1, and the value of i
is printed. Once i
becomes 6, the condition becomes false, and the loop stops.
Controlling Loop Execution with Conditions
The term “Controlling Loop Execution with Conditions” refers to the use of conditional statements within a loop to determine whether to continue iterating or to terminate. In simple terms, it’s about deciding when to start or stop a loop based on specific parameters.
For example, if you have a loop that iterates through the numbers 1–10. You might want to print just even numbers. In this case, you would use a condition (such as an if statement) inside the loop to determine whether the current number is even. If it is, you would do the appropriate action (for example, printing the number); otherwise, you would skip that iteration of the loop.
Controlling loop execution with conditions allows you to make your code more flexible and responsive to various conditions by changing the loop’s behavior based on specific requirements.
Example 2: Using For Loops to Iterate Through Arrays
You can iterate through each element of an array in C programming by using for loops. Arrays are collections of the same type of variables stored in continuous memory regions. Using for loops allows you to efficiently retrieve each element of an array one at a time.
In this example, we will show how to use for loops to loop through arrays in C programming.
#include <stdio.h>
int main() {
// Define an array of integers
int numbers[] = {1, 2, 3, 4, 5};
// Calculate the length of the array
int length = sizeof(numbers) / sizeof(numbers[0]);
// Iterate through the array using a for loop
printf("Array elements: ");
for (int i = 0; i < length; i++) {
printf("%d ", numbers[i]);
}
return 0;
}
Output:
Array elements: 1 2 3 4 5
Explanation:
- We create an array called numbers with integer values.
- To get the length of the array, divide its entire size by the size of a single element.
- Using a for loop, we iterate through the array and print each element to the the console.
- The loop goes from index 0 to length -1, ensuring that all elements are accessible and printed.
- Finally, we display the array elements, separated by spaces.
Nested For Loops: Understanding Multiple Levels of Iteration
Nested for loops in C allow you to have loops inside other loops. It’s like having a loop within a loop.
Assume you’re creating a grid of squares in a game. You would use a for loop to move horizontally, followed by another for loop to move vertically. Each combination of horizontal and vertical movement results in a square in the grid.
Similarly, in programming, you can use nested for loops to complete tasks that need multiple levels of iteration. For example, if you had a two-dimensional array (such as a grid or table), you may use nested for loops to access and manipulate each element in the array.
Every time the outer loop runs, the inner loop completes. So, if the outer loop runs three times and the inner loop runs four times, the inner loop will execute four times for each outer loop iteration, for a total of twelve executions.
Just remember to keep track of your loop counters and conditions to avoid infinite loops and ensure your code works properly.
Example 3: Nested For Loop Application
In this example, we will look at a practical use of nested for loops in C programming. Nested loops are mostly used when working with multidimensional arrays or when repetitive operations must be done on each element of a collection.
#include <stdio.h>
int main() {
int rows, cols;
printf("Enter the number of rows: ");
scanf("%d", &rows);
printf("Enter the number of columns: ");
scanf("%d", &cols);
// Nested for loop to print a rectangular pattern
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("* ");
}
printf("\n");
}
return 0;
}
In this code, we ask the user to specify the number of rows and columns for a rectangular design. Then, using nested for loops, we run through each row and column, printing asterisks (*) in a rectangle pattern.
This example shows how nested for loops may be used to efficiently handle two-dimensional structures such as matrices and grids.
Tips and Best Practices for Using For Loops Effectively
- Keep It Simple: To avoid confusion, keep your loop’s logic clear.
- Select Descriptive Variable Names: Use meaningful variable names to boost readability.
- Avoid Infinite Loops: Make sure your loop’s conditions allow for termination.
- Initialize variables Properly: Before the loop begins, initialize its control variables.
- Use Increment/Decrement Wisely: Make that your increment/decrement logic aligns with the loop’s objective.
- Check for Off-by-One Errors: To avoid unexpected behaviour, ensure that your loop’s condition and control variables are correctly aligned.
- Minimize computation inside the loop: If a calculation does not need to be repeated, move it outside the loop.
- Consider loop optimization: Sometimes a different looping strategy can enhance performance.
- Keep Code DRY (Don’t Repeat Yourself): If you find yourself duplicating code within the loop, think about rewriting it into a different function.
- Test and Debug: Before deploying your code, thoroughly test your loop and debug any errors that arise.