Understanding tokens in C programming is analogous to studying the alphabet before writing a story. Tokens are the essential units of code that make up the language’s syntax. In this lesson, we’ll walk you through the various types of tokens in C, explaining why they’re important and how they help to develop robust and efficient programs. Whether you’re a beginner looking to learn the fundamentals or an experienced programmer looking to improve your comprehension, this complete exploration will provide you with the knowledge you need to confidently navigate C programming. Let’s get started!
Table of Contents
What are Tokens?
Tokens are important in C programming. Tokens are the smallest units in a C program and represent individual elements including keywords, identifiers, constants, operators, and special symbols. They act as code building blocks, providing the syntax and structure required for the program to function.
Tokens are classified into several categories, each providing a unique purpose in the program’s execution. Keywords in C are reserved words with predetermined meanings, whereas identifiers are names assigned by the user to variables, functions, and other things. Constants represent constant values, whereas operators operate on operands. Punctuation and other characters used for syntax and organization are examples of special symbols.
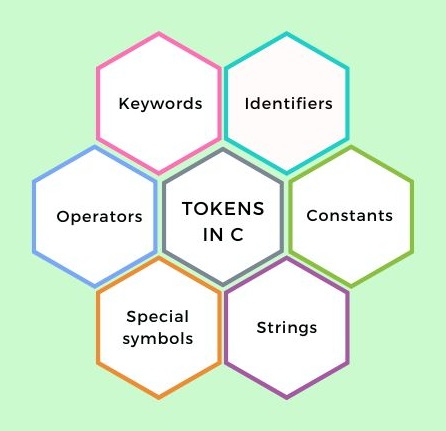
Importance of Understanding Tokens in C
A solid understanding of tokens is required for building proper and efficient C programs. Programmers can precisely describe logic, manipulate data, and regulate program flow if they recognize and use tokens efficiently. Furthermore, understanding tokens allows engineers to debug issues and improve code performance. Token mastery enables programmers to develop clean, simple, and maintainable code, making it a must-have talent for anyone getting started with C programming.
Types of Tokens in C Programming
- Keywords:
- Keywords are preset reserved terms in C programming that have specific meanings but cannot be used as identifiers.
- Keywords include integer, float, if, for, while, return, and so on.
- Identifiers:
- Identifiers are names assigned to variables, functions, arrays, and so on by the programmer.
- Identifiers must follow specific standards, such as beginning with a letter (or underscore) and include letters, digits, and underscores.
- Identifiers can be variable names like count, function names like calculateTotal, or array names like myArray.
- Constants:
- Constants are constant values that do not change during a program’s execution.
- Constants can be classified as integer, floating-point, character, or string literals.
- Integer constants are written as 10, 0xFF, etc., but floating-point constants are represented as 3.14, 2.0e-3, etc.
- Strings:
- Strings are collections of characters enclosed in double quotation marks (“).
- In C programming, strings are represented as arrays of characters terminated with a null character (‘\0’).
- String examples include “Hello, World!”, “C programming”, and “1234”.
- Operators:
- Operators are symbols that perform operations to operands.
- The C programming language offers a variety of operators, such as arithmetic (+, -, *, /), relational (<, >, ==,!=), and logical (&&, ||,!).
- Special symbols:
- Punctuation marks, braces, parentheses, commas, semicolons, and other special symbols are used in C programming to indicate syntax and control flow.
- Examples of special symbols are {, }, (,),,, ;, and more.
Lexical Analysis: How Tokens are Recognized
The first step of the compilation process is lexical analysis, which examines the source code and converts it into meaningful tokens for subsequent processing by the compiler. Lexical analysis in C programming is the process of recognizing individual code components known as tokens.
Here’s how lexical analysis recognises tokens:
- Scanning: The source code is scanned character by character, left to right.
- Tokenization: During scanning, sequences of characters are combined to produce tokens according to established criteria. These rules specify the syntax and structure of valid tokens in C programming.
- Classification: Tokens are divided into several categories, including keywords, identifiers, constants, operators, strings, and special symbols.
- Token Output: After classification, recognized tokens are transferred to the compiler’s next phase for additional processing, such as parsing and semantic analysis.
For example, consider the C code snippet int main() { return 0; }
. During lexical analysis, the following tokens would be recognized:
- Keyword:
int
- Identifier:
main
- Special symbol:
(
- Special symbol:
)
- Special symbol:
{
- Keyword:
return
- Constant:
0
- Special symbol:
;
- Special symbol:
}
Overall, lexical analysis is essential in breaking down source code into manageable units (tokens) that the compiler can then analyze and transform into machine-readable code.