Logical operators are effective tools for making condition-based decisions in C programming. They help in evaluating expressions and determining whether they are true or untrue. The logical operators NOT (!), OR (||), and AND (&&) are examples. Understanding the operation of these operators is essential for managing the program’s flow and efficiently using conditional expressions. This guide will provide a clear and basic explanation of logical operators, allowing beginners to better understand their ideas and applications in C programming.
Table of Contents
Logical AND Operator (&&)
The logical AND operator (&&) in C is used to combine two conditions. It returns true when both conditions are met; otherwise, it returns false.
Truth Table:
The truth table for the Logical AND operator is as follows:
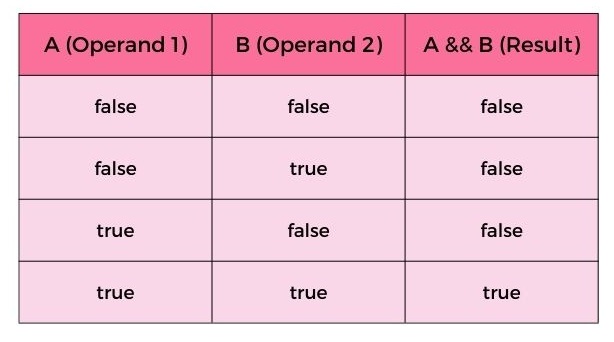
- When both operands are true, the result is true.
- Otherwise, the result is false.
Example:
#include <stdio.h>
int main() {
int x = 5;
int y = 7;
// Using logical AND operator
if (x > 0 && y > 0) {
printf("Both x and y are positive.\n");
} else {
printf("At least one of x or y is not positive.\n");
}
return 0;
}
In this example:
x
is 5, andy
is 7.- The condition
x > 0
is true becausex
is positive. - The condition
y > 0
is also true becausey
is positive. - Since both conditions are true, the logical AND operator (
&&
) evaluates to true, and the statement inside theif
block is executed, printing “Both x and y are positive.”
Logical OR Operator (||)
The logical OR operator (||) in C is used to perform a logical OR operation between two conditions. It returns true if at least one of the conditions is true; otherwise, it returns false.
Truth Table:
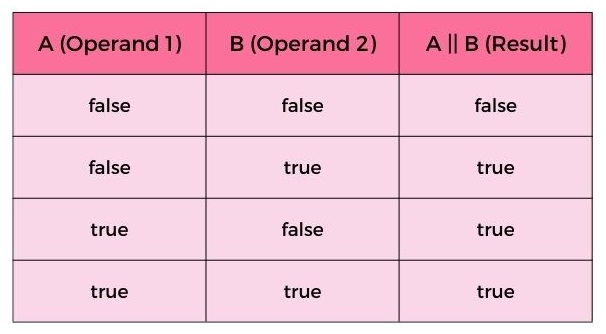
- If both expressions are false, the result is false.
- If one of the expressions is true, the result is true.
- Even if both expressions are true, the result is still true.
Example:
#include <stdio.h>
int main() {
int x = 5;
int y = 10;
// Using Logical OR operator
if (x < 4 || y > 8) {
printf("At least one condition is true.\n");
} else {
printf("Both conditions are false.\n");
}
return 0;
}
In this example, x < 4
evaluates to false because 5 is not less than 4, and y > 8
evaluates to true because 10 is greater than 8. Since at least one condition is true, the message “At least one condition is true.” will be printed.
Logical NOT Operator (!)
The Logical NOT Operator, represented by the symbol “!,” is a unary operator in C that negates or reverses the truth value of its operand. Simply put, it changes the value of a condition from true to false or vice versa.
Truth Table:
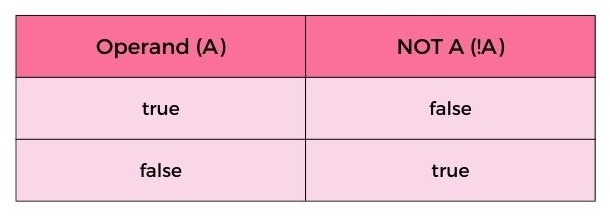
- If the operand is true (non-zero), the logical NOT operator will return false (0).
- If the operand is false (zero), the logical NOT operator will return true (1).
Example:
Let’s say we have a variable isRainy
that indicates whether it’s raining:
#include <stdio.h>
int main() {
int isRainy = 1; // Assume it's raining (true)
// Using Logical NOT Operator to check if it's not raining
if (!isRainy) {
printf("It's not raining.\n");
} else {
printf("It's raining.\n");
}
return 0;
}
In this example:
- Initially,
isRainy
is assigned the value 1, indicating true (it’s raining). - The
if (!isRainy)
statement is evaluated. SinceisRainy
is true (1),!isRainy
evaluates to false (0), meaning it’s not raining. - Thus, the output will be: “It’s raining.”
If we change the value of isRainy
to 0 (false), the output will be: “It’s not raining.”
Precedence and Associativity of Logical Operators
Precedence and associativity are programming notions that determine the order in which operators are evaluated in an expression. In C, logical operators such as AND (&&), OR (||), and NOT (!) follow the same rules.
- Precedence: When an expression contains more than one operator, the order in which they are evaluated is determined by precedence. Higher precedence operators are evaluated first.
In C, the precedence of logical operators is as follows, from highest to lowest:
- Logical NOT (
!
) - Logical AND (
&&
) - Logical OR (
||
)
For example, in the expression A && B || C
, the &&
operator has higher precedence than ||
, so A && B
is evaluated first, then the result is combined with C
.
- Associativity: When multiple operators with the same precedence appear in an expression, their order of evaluation is determined by associativity. It may be left-to-right or right-to-left.
Logical operators in C are left-to-right associative, which means they are evaluated from left to right.
For example, the expression A && B && C is evaluated from left to right. A && B are first examined, and the result is combined with C.