Welcome to the article “Mastering PHP Switch Case: A Comprehensive Guide.” If you’ve ever wondered how to efficiently manage many conditions in your PHP code, you’ve come to the correct spot. Switch case statements are a handy tool for easily managing a variety of scenarios. In this comprehensive book, we’ll walk you through the fundamentals to advanced techniques, with clear explanations and practical examples along the way. By the conclusion, you’ll be able to confidently use switch case statements in PHP applications, streamlining your code and improving your programming skills. Let’s get started!
Table of Contents
What is a switch case statement?
A switch case statement is a computer control structure that allows you to run different blocks of code depending on the value of a variable or expression. It offers an alternative to using multiple if…elseif…else statements when a single variable has multiple possible values.
In PHP, the switch case statement evaluates the value of an expression and then executes the code block associated with the matching case label. If none of the cases match, you can define a default case for execution.
Why use switch case in PHP?
- Readability: Switch case statements can help make your code more understandable and maintained, especially when there are several situations to consider.
- Simplicity: It reduces code complexity, especially when dealing with a large number of alternative conditions, by reducing nested if…elseif…else lines.
- Performance: Switch case statements can sometimes be more efficient than multiple if statements, especially when the evaluated expression has a limited range of possible values.
- Logical Structure: Switch case statements communicate conditional logic in a way that is simple and straightforward, making it easy to understand how your code flows.
- Familiarity: Switch case statements are a standard feature in many programming languages, thus using them in PHP can help developers who are already familiar with other languages understand your code more easily.
Basic Structure of Switch Case
In PHP, the switch case statement is an efficient way to compare a variable to many possible values. A switch case statement has the following components:
switch (variable) {
case value1:
// Code to be executed if variable equals value1
break;
case value2:
// Code to be executed if variable equals value2
break;
// Additional cases as needed
default:
// Code to be executed if variable doesn't match any case
}
- The switch keyword begins the switch case statement, which is followed by the variable being evaluated.
- Each case specifies one possible value for the variable.
- If the variable’s value matches one of the cases, the code block corresponding to that case is run.
- The break statement ends the switch case block, preventing code execution from moving on to the next case.
- The default case is optional and is used when none of the other cases match the variable’s value.
How Switch Case Works in PHP
- When a switch case statement is encountered, PHP evaluates the expression specified in the switch statement.
- It then compares the expression’s value to the case values.
- If a match is discovered, the associated block of code is executed, and the switch case statement ends (unless a break statement is omitted).
- If no matches are discovered, the code from the default case (if any) is executed.
- If no match is detected and there is no default case, the switch case statement exits without taking any further action.
- Switch case statements are a compact and effective technique to manage multiple conditional branches in PHP code, which improves readability and maintainability.
Demonstrating switch case with basic example
<?php
$day = "Monday";
switch ($day) {
case "Monday":
echo "It's Monday!";
break;
case "Tuesday":
echo "It's Tuesday!";
break;
case "Wednesday":
echo "It's Wednesday!";
break;
default:
echo "It's not Monday, Tuesday, or Wednesday.";
}
?>
Explanation:
- In this example, we have assigned the variable $day to “Monday”.
- The switch statement compares the value of $day to each condition.
- If $day matches one of the cases, the code block that corresponds to it is executed.
- After the matched case has been executed, the switch block is exited using the break statement.
- If no case matches, the code inside the default block executes.
Handling multiple conditions within a single case
PHP allows you to handle multiple conditions within a single case block by using the case keyword followed by several values separated by commas. This allows you to run the same block of code with multiple values.
Example:
$day = "Monday";
switch ($day) {
case "Monday":
case "Tuesday":
case "Wednesday":
case "Thursday":
case "Friday":
echo "Weekday";
break;
case "Saturday":
case "Sunday":
echo "Weekend";
break;
default:
echo "Invalid day";
}
Because each weekday is mentioned sequentially without a break statement, the identical code block executes from Monday to Friday. The break statement follows the block to prevent fallthrough to the next instance.
Using Break and Fallthrough Effectively
- Break Statement: Exit the switch case statement when a condition is met. Without it, the execution would proceed to the next case regardless of whether the condition was true or false.
- Fallthrough: Fallthrough happens when the break statement is absent, forcing execution to proceed to the next case block. While fallthrough is sometimes purposeful, using break to explicitly regulate the flow of execution is typically regarded best practice.
$option = "B";
switch ($option) {
case "A":
echo "Option A selected";
break;
case "B":
echo "Option B selected";
// No break statement, falls through to the next case
case "C":
echo "Option C selected";
break;
default:
echo "Invalid option";
}
If $option is “B”, both “Option B selected” and “Option C selected” will be echoed because there is no break statement following the “Option B selected” case block.
Understanding how to handle multiple cases and efficiently use break statements will allow you to develop more efficient and maintainable switch case statements in PHP.
Exploring the Default Case Option
In PHP switch case statements, the default case is used as a fallback option. It is executed whenever none of the stated case criteria match the switch expression.
When to Use the Default Case
- Use the default case to handle any unexpected or unspecified conditions.
- It serves as a fallback alternative when none of the specified conditions are met.
How to Implement the Default Case
- Place the default case at the conclusion of the switch statement, following all other cases.
- It does not require a condition because it is only run if none of the preceding cases are met.
Example:
$day = "Sunday";
switch ($day) {
case "Monday":
echo "It's Monday!";
break;
case "Tuesday":
echo "It's Tuesday!";
break;
// More cases...
default:
echo "It's neither Monday nor Tuesday...";
}
In this example, if $day
is not equal to any of the specified cases, the default
case will be executed, providing a fallback message.
Use the default case to notify users or handle situations where the required conditions are not met. Give meaningful feedback or instructions within the default case to help users or developers. While the default case is optional, it is generally considered good practice to include it for thorough error handling. Ensure that the default case handles unusual scenarios appropriately to avoid silent failures or misunderstanding.
Comparison of switch case with if…else statements
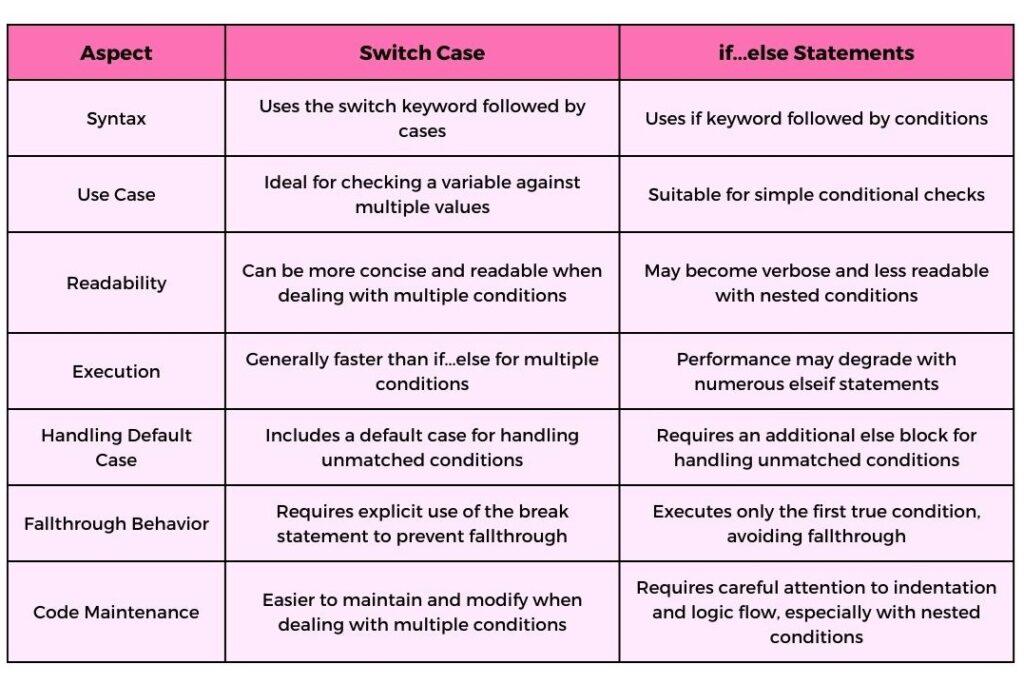
When choosing the right control structure
Use the Switch Case:
- When dealing with a single variable with several possible values.
- For situations where clarity and readability are critical.
- When performance optimisation is critical, especially for large sets of criteria.
Use If…Else/Elseif:
- For complex conditions that include several variables or expressions.
- When flexibility in condition evaluation order is required.
- Switch case behaviour may differ between PHP versions, so preserving backward compatibility with earlier PHP versions is required.