Welcome to our complete tutorial on understanding storage classes in C! Understanding storage classes is essential for any new programmer or experienced developer trying to improve their understanding of C. In this book, we will look at the core ideas of storage classes, including how they control the visibility, scope, and lifetime of variables in C programs.
We’ll go over everything from the well-known auto and static storage classes to the lesser-known extern and register functions. By the end of this guide, you will have a solid understanding of when and how to use each storage class in C programming. Let’s explore in and solve the secrets of C storage classes together!
Table of Contents
What are storage classes?
Storage classes in C specify the scope, visibility, and lifetime of variables and functions in a program. They control where and how variables and functions are kept in memory during program execution.
Why are they important in C programming?
Storage classes are critical in C programming because they manage memory and govern the behavior of variables and functions. They contribute to memory optimization, effective variable initialization, scope and visibility management, and efficient code organization. Understanding storage classes is critical for developing efficient, dependable, and maintainable C programming.
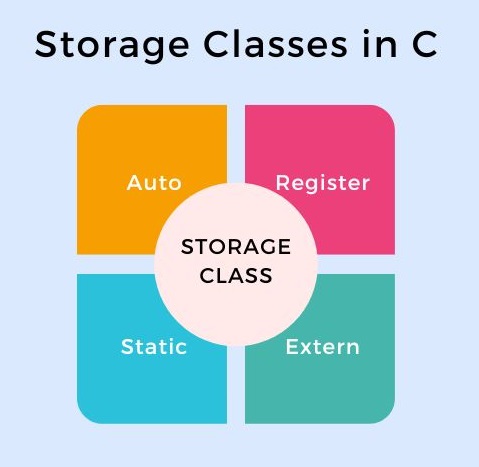
Auto Storage Class
In C programming, variables are often categorized into storage classes based on their scope, lifetime, and accessibility. The automatic storage class is one such classification, which is frequently expressed by the auto keyword.
Automatic variables are ones that are declared within a function or block and do not have a storage class specified. They are created when the function or block executes and destroyed when it terminates.
Scope and Lifetime
The scope of automatic variables is restricted to the block or function in which they are declared. They cannot be accessible from outside of the block or function. In addition, their lifetime is limited to the duration of the block or function execution. When the block or function leaves, the memory allocated for automatic variables is released.
Examples and Use Cases
#include <stdio.h>
void exampleFunction() {
auto int num = 10; // Declaration of automatic variable
printf("Automatic variable num: %d\n", num);
// Other code here...
}
int main() {
exampleFunction();
// num variable is not accessible here
return 0;
}
In this example, num is declared as an automatic variable within the exampleFunction() function. It can only be accessed from the exampleFunction() and not from the main() function or any other portion of the program.
Automatic variables are frequently used to store temporary data or intermediate results within functions or blocks. If the programmer does not explicitly initialize them, they are defaulted to garbage values. Automatic variables are lightweight and can be used in situations where their scope and lifetime match the program logic’s requirements.
Register Storage Class
In C programming, the register storage class is used to specify which local variables should be saved in CPU registers rather than RAM. These variables are accessed faster because data is read from registers rather than memory. It is important to remember, however, that the register keyword is only a hint to the compiler, and a variable declared with register will not necessarily be put in a register.
Register variables are defined by using the register keyword followed by the data type and variable name, just as other variable declarations. For example:
register int count;
Benefits and Limitations
Benefits:
- Faster Access: Register variables are stored in CPU registers, which results in faster access times.
- Performance Enhancement: Especially effective in time-sensitive situations where performance optimization is essential.
Limitations:
- Limited Number: The number of variables that can be stored in registers is restricted, and the compiler may opt not to allocate a register for a variable even if it is declared with the register keyword.
- Size Restrictions: Because register variables do not have memory addresses, they cannot be used with the unary & (address of) operator.
- Compiler Discretion: If the compiler determines that putting the variable in a register will not increase speed, the register keyword may be ignored.
When to Use Register Variables
Register variables should be used carefully, especially in performance-critical areas of code where speed optimization is required. However, it is critical to trust the compiler’s judgement and use register variables where their benefits are likely to be realized.
Example:
#include <stdio.h>
int main() {
register int i;
int sum = 0;
for (i = 1; i <= 1000; ++i) {
sum += i;
}
printf("Sum of numbers from 1 to 1000: %d\n", sum);
return 0;
}
In this example, the variable i is declared as a register variable inside the loop. Since the loop iterates frequently, storing i in a register may result in speed advantages. However, if the compiler determines that placing i in a register will not greatly improve speed, it may ignore the register keyword.
Static Storage Class
In C programming, the static storage class is used to declare variables and functions that will have a fixed memory allocation throughout the program’s execution. This means that their memory is allocated once and remains for the duration of the programme.
Static Variables: Initialization and Use:
Static variables are only initialized once and keep their values throughout function calls. They keep their values even after the function in which they are declared terminates. This makes static variables important for preserving state between function calls.
Scope and lifecycle of static variables:
Static variables have a limited scope within the block in which they are defined, but their lifetime spans the entire program’s execution. This implies they are only available within the block in which they are defined, but their value remains constant throughout the programme.
Static Functions: Benefits and Useful Applications
Static functions are functions whose scope is confined to the file where they are defined. They cannot be accessed from outside the file. This encapsulates and organizes code. Static functions are frequently used when a function is only required within a single file and should not be available from other files.
Example:
#include <stdio.h>
// Function declaration
void incrementCounter();
int main() {
// Calling the function multiple times
incrementCounter();
incrementCounter();
incrementCounter();
return 0;
}
void incrementCounter() {
// Static variable declaration and initialization
static int counter = 0;
// Incrementing the static variable
counter++;
// Printing the value of the static variable
printf("Counter: %d\n", counter);
}
In this example, the incrementCounter() function includes a static variable counter. Each time the function is invoked, the counter variable retains its previous value and increases by one. The result will be:
Counter: 1
Counter: 2
Counter: 3
This demonstrates how static variables keep their values between function calls and have a lifespan that beyond the function’s scope.
Also Read : Simplified Guide to Logical Operators in C Programming
Extern Storage Class
In C programming, the extern storage class specifier is used to declare a variable or function from another source file. It’s similar to telling the compiler, “Hey, this thing exists, but its actual definition is somewhere else.”
Extern Variables: Declaring and Accessing:
When you declare a variable with extern, you inform the compiler that the variable is declared elsewhere and that its definition will be provided by another file during the linking phase of compilation. You do not allocate memory for this variable in the current file; instead, you state, “Trust me, it’s defined somewhere else.”
Linkage and Visibility:
The extern keyword also addresses linking and visibility. Linkage refers to how a variable is related to other files during the linking process. Visibility impact whether a variable can be accessed from other files or only from the current file.
Extern with Functions: Implementation and Benefits.
Similarly, you can combine extern and functions. When you declare a function using extern, you inform the compiler that it is defined in another source file and will be linked later. This is useful when you have functions defined in numerous files but wish to access them from a separate one.
Example:
Let’s imagine you have two source files: main.c and helper.c.
In helper.c you have:
// helper.c
#include <stdio.h>
int globalVar = 10;
void helperFunction() {
printf("This is a helper function.\n");
}
Now, in main.c
, you want to use the globalVar
and helperFunction
defined in helper.c
. You’ll declare them as extern
:
// main.c
#include <stdio.h>
extern int globalVar; // Declaration of globalVar
extern void helperFunction(); // Declaration of helperFunction
int main() {
printf("Global variable: %d\n", globalVar);
helperFunction();
return 0;
}
When you compile and link both files together, the linker will resolve references to globalVar and helperFunction in main.c to their definitions in helper.c, allowing your program to execute smoothly.