PHP functions are critical building blocks in web development, allowing developers to create reusable code for specific tasks. They provide a method for effectively grouping code, enhancing readability, and reducing redundancy. This section will cover the foundational concepts of PHP functions, including their useful uses in web development projects and their basic structure. Whether you’re new to PHP or want to improve your knowledge, this article will help you understand the concept of functions. Now let’s get started!
Table of Contents
What are PHP Functions?
PHP functions are little helpers in your programming. Imagine you have a big task to complete, such as baking a cake. Instead of doing everything yourself, you can divide it into smaller parts. Each of these subtasks can be handled by a function.
A function in PHP is a group of instructions used to perform out a certain task. To bake a cake, for example, you may have a function called “makeCake()” that covers all the necessary steps, such as mixing ingredients, baking in the oven, and decorating.
Functions in PHP help you organize your code more effectively. Instead of writing the same code repeatedly, you can write it once inside a function, and then simply call that function whenever you need to do that. It helps you organize your code more easily and saves time.
PHP functions are like a cookbook for executing tasks in your code. You define them once, and you can use them whenever you need to do that specific task.
Advantages of Using Functions in PHP
Using functions in PHP offers several advantages that make coding easier, more organized, and efficient.
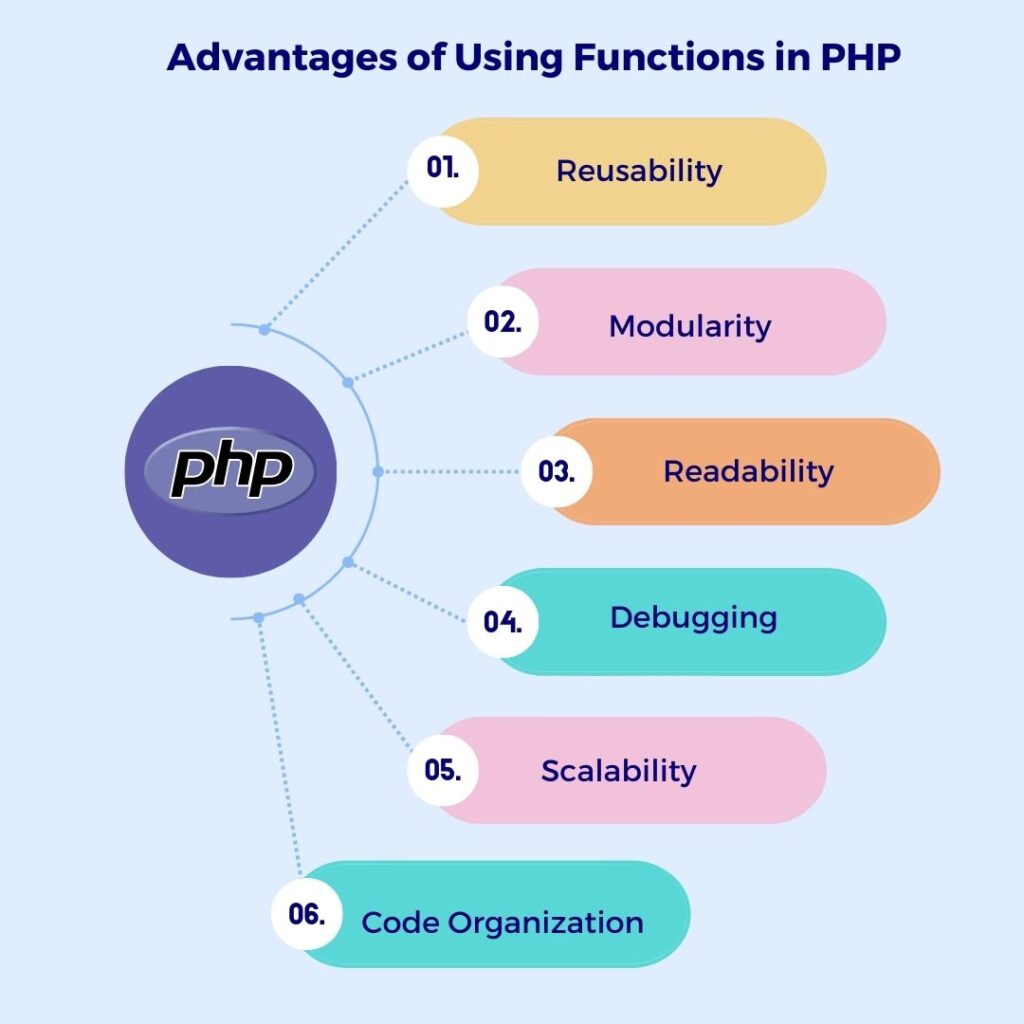
- Reusability: Functions enable you to write a single block of code and reuse it several times in your program. Instead than writing the same code again and over, simply call the function whenever you need it.
- Modularity: Functions help you to divide your code into smaller, more manageable portions. Each function performs a specific task, making your code easier to understand and maintain. This modular architecture encourages code reusability and collaboration among developers.
- Readability: You may improve the readability and understanding of your code by giving your functions meaningful names. Because functions are self-contained blocks of code, they make it simpler for future developers—or even for you—to understand what each section of the code does.
- Debugging: When your code is divided into functions, it is easier to find and correct issues. You may isolate the issue function and focus on debugging that specific section of code without having to go through the full program.
- Scalability: As your project expands, functions let you scale your codebase more effectively. You can add new features or adjust old ones by simply creating or updating functions, without having to completely rewrite the codebase.
- Code Organization: Functions help you to logically organize your code. Grouping related tasks into different functions allows you to maintain a clear structure in your codebase, making it easier to navigate and manage.
Anatomy of a PHP Function
A PHP function is similar to a little program contained within a larger program. It’s a collection of instructions that you can reuse in your code repeatedly without having to write them from scratch. Let us divide it down into parts:
- Function Name: A function has a name, just like a person. This is what you’ll use to call the function later in your code.
- Parameters (Optional): Consider parameters as variables that the function can use. They act as inputs to the function, allowing it to understand what to operate with.
- Function Body: Magic happens in this area. Use curly brackets {} to specify the function’s instructions. These could be calculations, actions, or whatever else you wish your function to perform.
- Return Statement (Optional): Sometimes, you would like your function to return a result once it has completed its work. This is where the return statement comes in. It returns a value to the point in your code where the function was called.
Here’s a simple example:
// Function declaration
function greet($name) {
// Function body
$message = "Hello, $name!";
return $message; // Return statement
}
// Function call
echo greet("John"); // Outputs: Hello, John!
In this example:
greet
is the function name.$name
is a parameter.function greet($name) { ... }
is the function body.return $message;
sends back the greeting message.echo greet("John");
calls the function with “John” as the input and outputs the result.
Defining Functions in PHP
In PHP, a function is similar to a recipe or a set of instructions that performs a certain purpose. To define (create) a function, simply follow these steps:
1. Select a Name: Give a name for your function that describes what it does. For example, if your function adds two numbers, you could call it “addNumbers”.
2. Write the Function Header: Begin with the keyword function, followed by the name of your function, and then brackets (). Inside the brackets, you can optionally specify the arguments (inputs) that your function requires to perform its purpose. If your function does not require any parameters, leave the brackets empty.
Example with parameters:
function addNumbers($num1, $num2) {
Example without parameters:
function greet() {
3. Write the Function Body: This section contains the actual code that explains the functions’ operations. It could be anything from basic computations to complex operations. Enclose your function’s body in curly braces {}.
Example:
function addNumbers($num1, $num2) {
$sum = $num1 + $num2;
echo "The sum is: " . $sum;
}
4. End with a Closing Brace: Close your function with a curly brace after writing the code.
Example:
function addNumbers($num1, $num2) {
$sum = $num1 + $num2;
echo "The sum is: " . $sum;
}
That’s it! Once you’ve defined your function, you can use it (call it) whenever you need to perform the task it’s designed for.
Calling Functions in PHP
Calling functions in PHP is similar to using predefined tools to complete specific tasks in your code. Once you’ve defined a function, you can call it whenever you need to execute its instructions.
When you call a function in PHP, you are essentially telling the interpreter to run the code within that function. It is similar to requesting that PHP perform a specific action.
Example:
Let’s say you have a function called greet()
that simply echoes a greeting message.
<?php
// Define the function
function greet() {
echo "Hello, World!";
}
// Call the function
greet();
?>
In this example:
- Using the function keyword, we define a function called greet().
- There is just one line of code that echoes “Hello, World!” inside the function.
- To call the function, simply enter its name followed by brackets (greet();).
- When PHP detects welcome();, it moves to the greet() function, performs the code inside it (which echoes “Hello, World!”), and then returns to where it left off in the main code.
Parameters and Arguments in PHP Functions
Parameters and arguments in PHP functions are like ingredients and values used to make a recipe.
- Parameters: These are the variables specified in the function definition. They serve as placeholders for the values that will be supplied when the function is called.
- Arguments: These are the actual values supplied to the function when it is called to fill out the parameters.
Example:
Let’s say we have a function to calculate the area of a rectangle:
function calculateRectangleArea($length, $width) {
$area = $length * $width;
return $area;
}
- Here,
$length
and$width
are parameters. - When we call this function, we provide actual values for these parameters, which are called arguments:
$area_of_rectangle = calculateRectangleArea(5, 3);
- Here,
5
and3
are arguments passed to the function. - Inside the function,
$length
will take the value5
and$width
will take the value3
. - The function will calculate the area using these values and return the result.
Also Read : Exploring PHP Data Types: A Beginner’s Journey
Return Values in PHP Functions
What a function returns after it has completed its execution is known as a return value in PHP. It’s like the answer to a question. When you call a function, you may want it to return something that may be used later in your code.
Here’s a basic explanation with an example:
Let’s assume you have a function named addNumbers() that adds two integers together:
function addNumbers($num1, $num2) {
$sum = $num1 + $num2;
return $sum; // This is the return value
}
n this example, $sum
is the result of adding $num1
and $num2
. But instead of just printing it out, we use the return
keyword to send that value back to wherever the function was called from.
Now, when you call addNumbers()
and pass it two numbers:
$result = addNumbers(5, 3);
echo $result; // This will output 8
The function calculates the sum of 5 and 3 and returns the result, which is 8. Then, we store that result in the variable $result
and can use it elsewhere in our code. That’s how return values work in PHP functions! They let functions communicate their results back to the rest of your program.