Understanding variables is essential in the dynamic world of PHP development since it allows you to build robust and flexible programmes. Variables are containers for storing various types of data, such as numbers, text, or complex structures, allowing for dynamic and flexible coding. Learning to create, handle, and manage variables efficiently is crucial for any PHP developer, regardless of experience level.
Table of Contents
Introduction to PHP Variables
Variables are similar to containers in the world of PHP programming. They store several types of information that your programme requires to function. Variables hold this data, which your PHP programmes can work with, whether it’s a word, a number, or a whole paragraph.
In PHP, a variable is just a name assigned to data that can change or vary. Consider it like a label you place on a box to help you remember what’s inside. Variables may store a wide range of data, including text, integers, and more complicated structures like objects and arrays. This is what makes them so beautiful.
Understanding variables is critical because they allow PHP scripts to be dynamic and responsive. By using variables, you can store and change data based on various criteria or user input, rather than hard-coding set values into your code. This improves the scalability, adaptability, and stability of your code.
Basic Syntax for Declaring Variables
In PHP, declaring variables follows a simple syntax:
$variable_name = value;
Here’s a breakdown of the syntax:
- $: In PHP, a variable is indicated by the dollar sign ($). It indicates to the interpreter that what follows is the name of a variable.
- variable_name: This is where you give your variable’s name. In PHP, variable names can have any combination of letters, numbers, or underscores after the initial letter (_). Variable names are case sensitive.
- =: In PHP, the assignment operator is the equals symbol (=). It assigns the value on the right to the variable on the left.
- value: You want to keep this data in the variable. It could be a number, a string (put in quotes), a boolean value (true or false), or the output of an expression.
Here are some examples of variable declarations in PHP:
$name = "John"; // Assigns the string "John" to the variable $name
$age = 30; // Assigns the integer 30 to the variable $age
$is_student = true; // Assigns the boolean value true to the variable $is_student
// Assigns the result of the expression 5 * 3 to the variable $result
$result = 5 * 3;
Variable Types in PHP
In PHP, variables can hold various types of data.
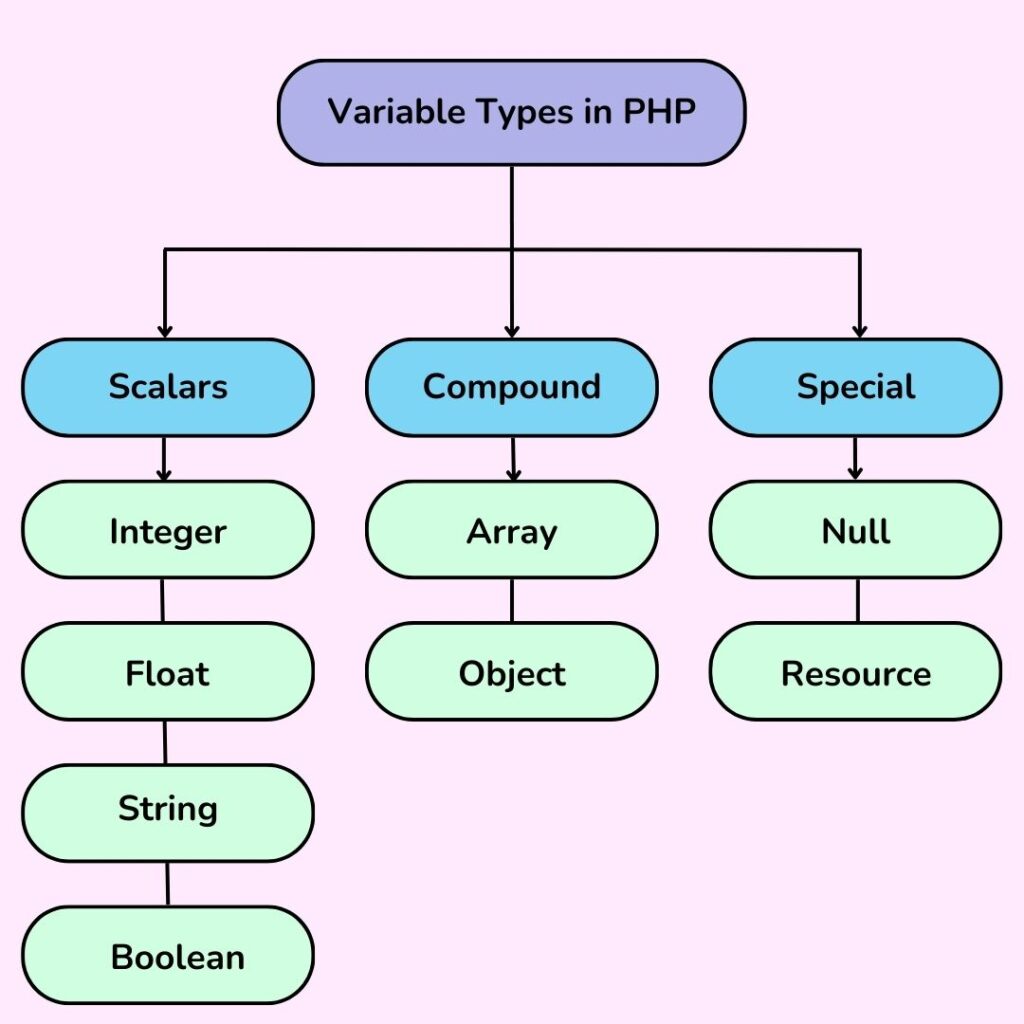
- Scalars: These are single-value data types.
- Integer: Whole numbers without decimal points, like 5 or -10.
- Float: Numbers with decimal points, such as 3.14 or -0.5.
- String: Textual data enclosed in single (”) or double (“”) quotes.
- Boolean: Represents true or false.
- Compound: These are data types that can hold multiple values.
- Array: A collection of key-value pairs or indexed values.
- Object: Instances of user-defined classes, encapsulating properties and methods.
- Special: These are unique types in PHP.
- Null: Represents the absence of a value.
- Resource: Holds a reference to an external resource, like a file or database connection.
Rules for naming variables in PHP
- Start with a letter or underscore.
- Followed by letters, numbers, or underscores.
- No spaces or special characters.
- Case-sensitive: $name and $Name are different.
- Choose descriptive names for clarity.
- Use camelCase or underscores_between_words for readability.
Variable Scope in PHP
In PHP, a variable’s scope defines where it can be accessed or changed within your code. PHP consists of three main scopes: local, global, and static.
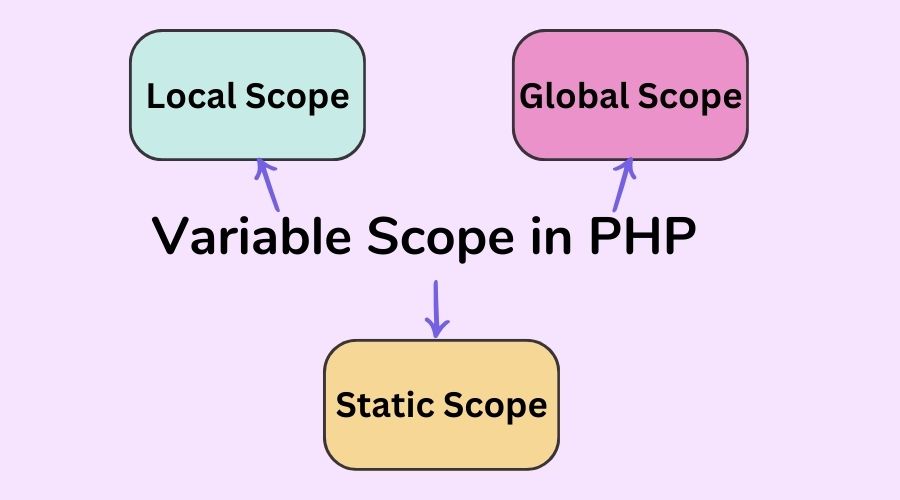
1. Local Scope:
Declared variables inside a function are regarded as local scope. They are only accessible through that function. These variables no longer exist after the code is executed.
function myFunction() {
$localVariable = "I am local";
echo $localVariable;
}
myFunction(); // Output: I am local
// echo $localVariable; // This will result in an error as $localVariable is not accessible outside the function
2. Global Scope:
Globally scoped variables are those that are defined outside of any class or function. They can be accessible from anywhere in the script, including inside functions.
$globalVariable = "I am global";
function myFunction() {
global $globalVariable;
echo $globalVariable;
}
myFunction(); // Output: I am global
echo $globalVariable; // Output: I am global
3. Static Scope:
Static variables in a function hold their value in between function calls. They are only initialised once and keep their value across multiple calls to the function.
function myStaticFunction() {
static $staticVariable = 0;
$staticVariable++;
echo $staticVariable;
}
myStaticFunction(); // Output: 1
myStaticFunction(); // Output: 2
myStaticFunction(); // Output: 3