Pointer arithmetic is a key technique in C programming that enables developers to perform tasks directly on memory addresses. Understanding how pointer arithmetic works is important for efficient and effective C coding because it allows greater control over data processing and memory allocation.
In essence, pointer arithmetic means performing mathematical operations on pointers to explore and manipulate the data they point to. This includes incrementing or decrementing pointers to move through arrays, adding or removing integers to navigate memory blocks, and even comparing pointers to determine relative positions in memory. These actions can greatly improve speed, particularly in systems programming, embedded systems, and applications that require smooth control of memory.
Pointers are a strong feature that allows for direct memory access, resulting in highly efficient and effective programs. Misuse of pointers, as well as incorrect pointer arithmetic, can lead to serious problems like memory leaks, segmentation faults, and data corruption. As a result, a strong understanding of pointer arithmetic not only improves a programmer’s ability to design efficient code, but also ensures the development of solid and reliable applications.
Table of Contents
Pointers in C
A pointer in C is a type of variable that holds the memory address of another variable. Instead of directly keeping a data value, a pointer holds the memory address where the data value is stored. This enables efficient data handling and memory management.
How Pointers Store Memory Addresses
When you declare a variable in C, the computer allocates a specific memory location to store its value. Every memory location has a unique address. This unique address is kept as a pointer, which allows you to indirectly access and change the value stored at that memory location.
For example, if you define an integer variable int x = 10;, the computer will store the value 10 at a specific memory address, such as 0x7fff5694. A pointer to this integer variable would have the address 0x7fff5694, not the value 10.
Pointer Declaration and Initialization
In C, you must declare and initialize pointers before using them. Here’s how it can be done:
Declaration: When you declare a pointer, you indicate the data type to which it points, followed by an asterisk (*) and the pointer name.
int *ptr;
In this example, ptr is an integer pointer. The asterisk (*) identifies ptr as a pointer variable.
Initialization: After declaring a pointer, you must initialize it with the address of a variable. To find a variable’s memory address, use the address-of operator (&).
int x = 10;
int *ptr = &x;
In this case, x is an integer variable with the value 10. The expression &x returns the memory address of x, which we assign to the pointer ptr. Now, ptr has the address of X.
To summarize:
The value of x is 10.
The address of x is stored in ptr.
Basic Pointer Operations
Dereferencing Pointers
Dereferencing a pointer refers to accessing the value stored at the memory address to which the pointer points. It’s the same as following an address to see what’s there.
Assume you have a residence address. The address does not indicate how the house looks inside. To see the actual residence, go to that address. In programming, the address is a pointer to a memory location, and the house is the value stored there.
Here’s how you dereference a pointer in C:
int number = 10; // A regular integer variable
int *ptr = &number; // A pointer variable that stores the address of 'number'
printf("%d\n", *ptr); // Dereferencing the pointer to get the value at that address (prints 10)
In this example, *ptr is used to get the value at the address stored in ptr.
Assigning values to pointers
Assigning a value to a pointer means that the pointer will store the address of a variable. This does not affect the variable’s value, but it does change the pointer’s destination.
Here is a simple example:
int number = 10; // A regular integer variable
int *ptr = &number; // A pointer variable that stores the address of 'number'
int anotherNumber = 20;
ptr = &anotherNumber; // Now 'ptr' points to 'anotherNumber'
printf("%d\n", *ptr); // Dereferencing 'ptr' now gives 20 (the value of 'anotherNumber')
Initially, ptr pointed to a number, but after the assignment, it pointed to another number.
Accessing Memory using Pointers
Pointers allow you to read from and write to specified memory locations directly. This is powerful, but it must be done correctly to avoid issues such as accessing incorrect memory addresses.
This is an example of reading and writing with pointers:
int number = 10;
int *ptr = &number; // Pointer to 'number'
// Reading the value at the memory location
printf("Value at ptr: %d\n", *ptr); // Outputs: 10
// Writing a new value to the memory location
*ptr = 20; // Changes the value of 'number' to 20
// Reading the new value
printf("New value at ptr: %d\n", *ptr); // Outputs: 20
printf("New value of number: %d\n", number); // Outputs: 20
In this example, *ptr = 20 modifies the value at the memory address indicated by ptr, which is the same as number. As a result, the number now has a value of 20.
Understanding Pointer Arithmetic
Pointer arithmetic is the process of performing mathematical operations on pointers. This is useful because it allows you to explore arrays and other data structures quickly. Pointers in C programming store variables’ memory addresses. Pointer arithmetic allows you to move from one memory address to another, which is extremely useful when working with arrays and dynamically allocated memory.
How Pointer Arithmetic Works in C
Pointers in C are used to refer to specific memory addresses. When you perform arithmetic on pointers, you go to different memory locations depending on the data type to which the pointer refers. For example, if you have a pointer to an integer (int) and you increment it, it will move to the next integer in memory rather than the next byte.
Incrementing and Decrementing Pointers
Incrementing Pointers: When you increment a pointer, it goes to the next element of the same type. For example, if ptr is an int pointer, ptr++ will transfer it to the next int in memory, skipping sizeof(int) bytes.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = arr;
ptr++; // Now ptr points to arr[1], which is 2
Decrementing Pointers: When you decrement a pointer, it goes to the previous element of its type. If ptr is pointing to arr[2], ptr– returns it to arr[1].
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = &arr[2];
ptr--; // Now ptr points to arr[1], which is 2
Adding and Subtracting Integers from Pointers
Adding Integers: When you add an integer to a pointer, it moves by the number of elements of that type. For example, ptr + 3 moves the pointer by three elements.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = arr;
ptr = ptr + 3; // Now ptr points to arr[3], which is 4
Subtracting Integers: Subtracting an integer from a pointer makes it to go backward by the number of elements.
int arr[5] = {1, 2, 3, 4, 5};
int *ptr = &arr[4];
ptr = ptr - 2; // Now ptr points to arr[2], which is 3
Example:
Here’s a simple example to illustrate pointer arithmetic:
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
int *ptr = arr; // ptr points to arr[0]
printf("Initial value: %d\n", *ptr); // Output: 10
ptr++; // Move to the next element
printf("After increment: %d\n", *ptr); // Output: 20
ptr = ptr + 2; // Move two elements ahead
printf("After adding 2: %d\n", *ptr); // Output: 40
ptr--; // Move back one element
printf("After decrement: %d\n", *ptr); // Output: 30
ptr = ptr - 1; // Move back one more element
printf("After subtracting 1: %d\n", *ptr); // Output: 20
return 0;
}
This example shows how the pointer ptr traverses the array arr using pointer arithmetic. This allows you to efficiently access different elements in the array without requiring array indices.
Pointer Arithmetic vs. Array Indexing
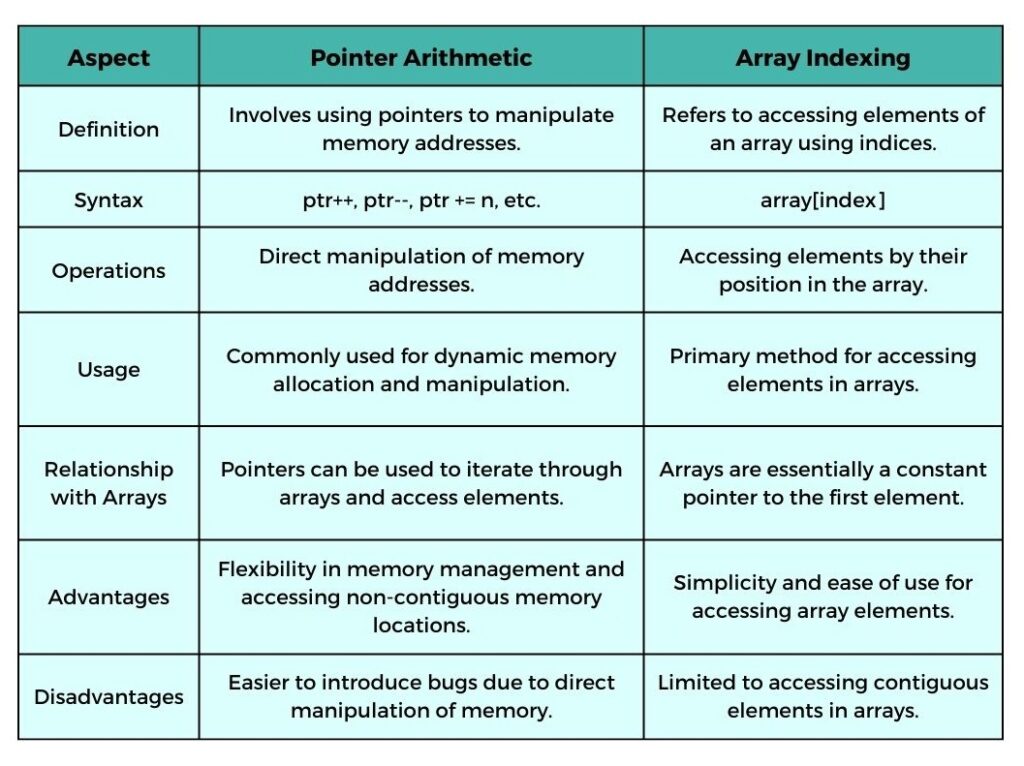