Understanding strings in C programming is like uncovering a hidden language within the code, and character arrays are at the core of this linguistic marvel. As the fundamental building blocks for text manipulation in C programming, strings—basically arrays of characters—play a crucial role.
In this blog article, we’ll delve into the complexities of strings in C, demystifying the potential hidden within character arrays. We’ll guide you through the fundamentals of working with strings, from string initialization to more complex manipulation techniques. This journey can help you learn more about string input/output, manipulation functions, comparison methods, and best practices—whether you’re a beginner programmer or looking to improve your C skills. Let’s explore the world of strings in C by discovering its mysteries.
Table of Contents
What are Strings in C?
Strings are collections of characters kept as arrays in the context of C programming. Unlike other programming languages, C doesn’t have a specialized string data type. Rather, strings are represented as arrays of characters that end with the null character ‘\0’. To handle strings properly, you must recognize this null character, which indicates the end of the string.
Any programmer should have a solid understanding of character arrays, which are the foundation of C strings. A linear sequence is formed by the correspondence between each character in the array and a certain string element. In C, manipulating and interpreting textual data efficiently is made possible by proficiency with character arrays.
Understanding character arrays is crucial since they are used frequently in C programs. For user interfaces, data processing, and input/output tasks, strings are essential. Programmers that know how to work with character arrays can do operations like concatenation, comparison, and modification with ease.
Fundamentals of Strings in C
Understanding Null-Terminated Strings
Character arrays are used to represent strings in C programming. The essential feature of C strings is that they are null-terminated, which means that the final character in a string is a special character known as the null character (‘\0’). This character indicates the end of the string and lets functions know how long it is.
Example:
char greeting[] = "Hello, World!"; // Null-terminated string
Declaring and Initializing Strings in C
There are several ways to declare and initialize strings in C. The most popular approach makes use of character arrays. Make sure you allocate enough space after declaring a string to accommodate the characters and the null terminator.
Example:
char welcome[20]; // Declaration without initialization
char message[] = "Greetings"; // Declaration with initialization
Accessing Individual Characters in a String
Using the character’s index in the array, one can retrieve specific characters in a string. Since C employs zero-based indexing, the first character is at index 0, the second is at index 1, and so on.
Example:
char word[] = "Programming";
char firstChar = word[0]; // Accessing the first character ('P')
char thirdChar = word[2]; // Accessing the third character ('o')
Character sequences kept in consecutive memory regions are called strings in C. An essential component that enables functions to identify the end of a string is the null terminator. Consider the required space for characters and the null terminator when declaring and initializing strings. To access characters, use index notation and remember the zero-based indexing rule.
String Input and Output
Reading Strings from the User
One of the most basic aspects of programming is reading user input. In C, the scanf function—or, more frequently, fgets—is used to read a string from the user.
Using ‘fgets
‘ for String Input:
- The command “fgets” (which stands for “file gets”) is frequently used to read a line of text from the keyboard’s standard input.
- Syntax: fgets(buffer, sizeof(buffer), stdin);
- buffer: A character array (string) where the input will be stored.
- sizeof(buffer): Indicates the buffer’s size in order to prevent overflows.
- Stdin: Stands for the keyboard’s standard input stream.
Example:
#include <stdio.h>
int main() {
char buffer[50]; // Assuming a buffer size of 50 characters
printf("Enter a string: ");
fgets(buffer, sizeof(buffer), stdin);
printf("You entered: %s", buffer);
return 0;
}
This code allows the user to enter a string, reads it with fgets, and then prints the entered string.
Using 'scanf
‘ for String Input:
- While scanf can be used for strings, it is generally less recommended because it might cause buffer overflow problems if not handled appropriately.
- Syntax:
scanf("%s", buffer);
"%s"
format specifier is used for strings.
Example:
#include <stdio.h>
int main() {
char buffer[50];
printf("Enter a string: ");
scanf("%s", buffer);
printf("You entered: %s", buffer);
return 0;
}
This code achieves the same result as the fgets
example, but be cautious with scanf
to avoid buffer overflow.
Displaying Strings to the User
In C, displaying strings is really simple. For this, the printf function is frequently utilized.
Using printf
for String Output:
- The
%s
format specifier is used to display strings.
#include <stdio.h>
int main() {
char greeting[] = "Hello, World!";
printf("Greetings: %s", greeting);
return 0;
}
This code declares a string greeting
and prints it using printf
.
String Functions
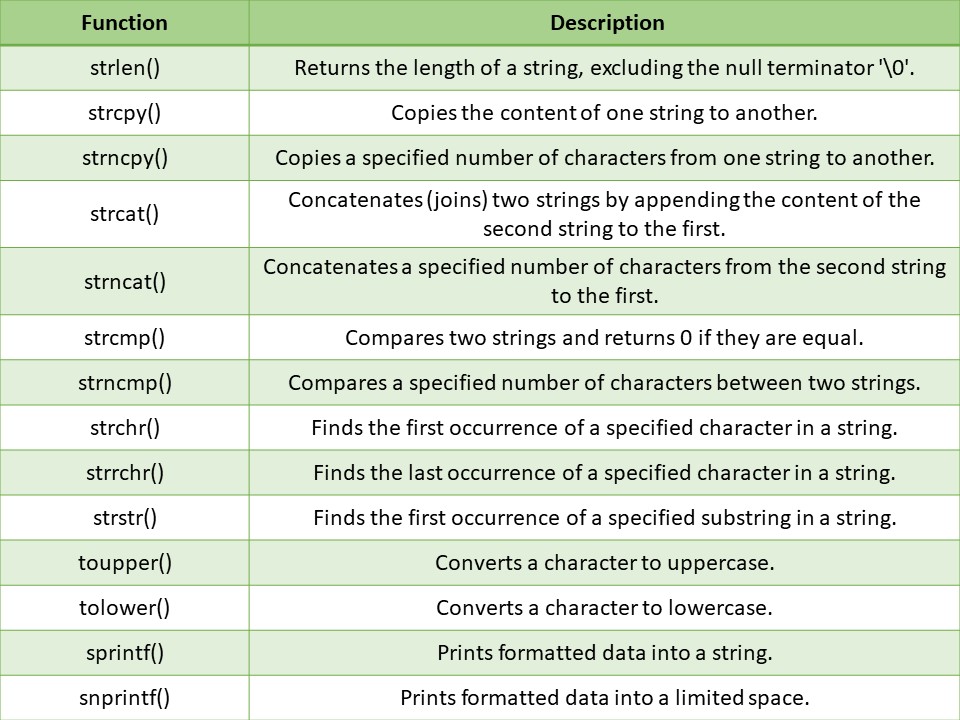
Examples of String Functions in C
strlen
()
Syntax:
size_t strlen(const char *str);
Example:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
size_t length = strlen(str);
printf("Length of the string: %zu\n", length);
return 0;
}
strlen()
returns the length of the stringstr
excluding the null terminator'\0'
.- In the example, the string “Hello, World!” has a length of 13 characters, so the output will be
Length of the string: 13
.
strcpy()
Syntax: char* strcpy(char *dest, const char *src);
Example:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[20];
strcpy(destination, source);
printf("Copied string: %s\n", destination);
return 0;
}
strcpy()
copies the contents of the source stringsrc
to the destination stringdest
.- In the example, the content of the
source
array is copied to thedestination
array, and the output will beCopied string: Hello, World!
.
strcat()
Syntex: char* strcat(char *dest, const char *src);
Example:
#include <stdio.h>
#include <string.h>
int main() {
char dest[20] = "Hello, ";
char src[] = "World!";
strcat(dest, src);
printf("Concatenated string: %s\n", dest);
return 0;
}
strcat()
concatenates (joins) the source stringsrc
to the end of the destination stringdest
.- In the example, the content of the
src
array is appended to thedest
array, and the output will beConcatenated string: Hello, World!
.
strstr()
Finds the first occurrence of a substring in a string.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char haystack[] = "The quick brown fox jumps over the lazy dog";
char needle[] = "brown";
char *result = strstr(haystack, needle);
if (result != NULL) {
printf("'%s' found at position %ld\n", needle, result - haystack);
} else {
printf("'%s' not found\n", needle);
}
return 0;
}
- The
haystack
is the string “The quick brown fox jumps over the lazy dog”. - The
needle
is the substring “brown”. - The
strstr
function is used to find the first occurrence of theneedle
in thehaystack
. - If the
needle
is found, the result is a pointer to the position of the first character of theneedle
in thehaystack
. The position is calculated by subtracting the base address ofhaystack
from the result pointer. - If the
needle
is not found, the function returnsNULL
.
strncpy()
It is used to copy a specified number of characters from one string to another.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[10]; // Destination buffer with a limited size
strncpy(destination, source, 5); // Copy the first 5 characters from source to destination
destination[5] = '\0'; // Manually null-terminate the destination string
printf("Copied string: %s\n", destination);
return 0;
}
In this example, only the first 5 characters of source
are copied to destination
, resulting in “Hello” being printed.