Operators are the fundamental units used in C programming that allow a program to perform out a variety of tasks and operations. If you’re new to programming or just getting started with the C language, the concept of operators may seem difficult at first. Don’t worry! In this guide, we’ll simplify and explain the principles of C operators.
In C, operators are symbols that carry out operations on values and variables. They are the building blocks of expressions and are essential for executing a wide range of operations, from fundamental arithmetic to more complex logical operations. Writing effective and useful C programs requires an understanding of operators.
In this article, we’ll look at a variety of operators such as arithmetic, assignment, comparison, logical, bitwise, and more. We hope to demystify C operators through simple explanations and practical examples, making them accessible and easy to understand for beginners. So let’s dive in and explore the world of C operators together!
Table of Contents
What are operators in programming?
Operators are characters or symbols used in programming that manipulate data. They are the fundamental components of expressions, allowing us to modify data in a variety of ways. Operators work with operands, which are data values or variables. These operations may involve mathematical computations, comparisons, logical operations, and others.
Importance of operators in C programming
Operators are important parts of the C programming language. They enable developers to handle data, manage programme flow, and conduct various computations. Understanding operators allows programmers to write code that is concise, readable, and efficient. Operators are essential in C programming, whether they do simple arithmetic or complex logical operations.
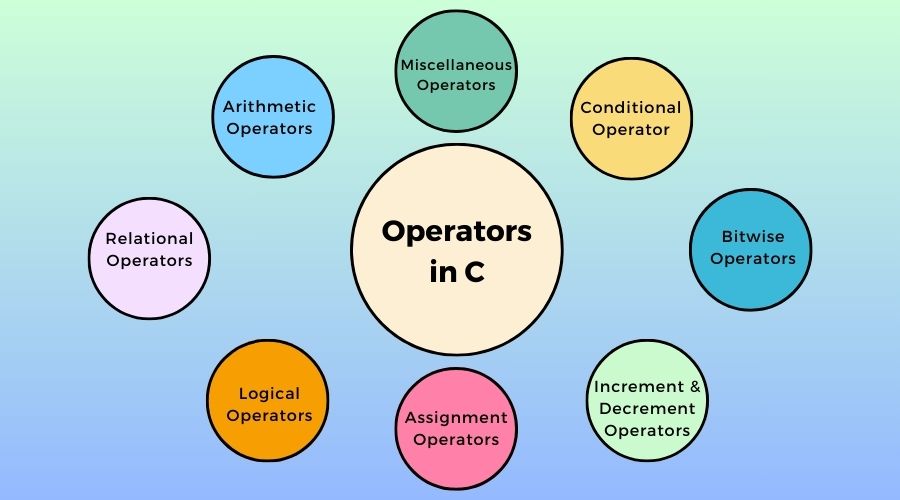
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations in C programming.
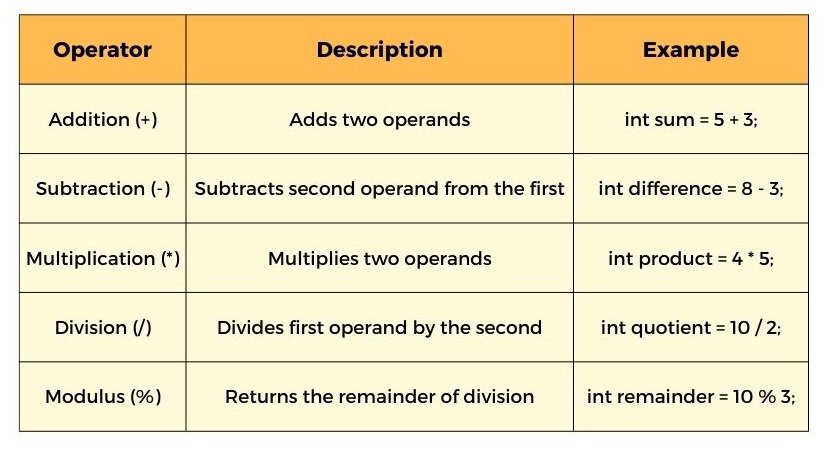
Example of using all arithmetic operators in C:
#include <stdio.h>
int main() {
int a = 10, b = 3;
int sum, difference, product, quotient, remainder;
// Addition
sum = a + b;
printf("Sum: %d\n", sum);
// Subtraction
difference = a - b;
printf("Difference: %d\n", difference);
// Multiplication
product = a * b;
printf("Product: %d\n", product);
// Division
quotient = a / b;
printf("Quotient: %d\n", quotient);
// Modulus
remainder = a % b;
printf("Remainder: %d\n", remainder);
return 0;
}
Output:
Sum: 13
Difference: 7
Product: 30
Quotient: 3
Remainder: 1
Relational Operators
Relational operators are used in C programming to compare two values and produce a logical result based on their relationship.
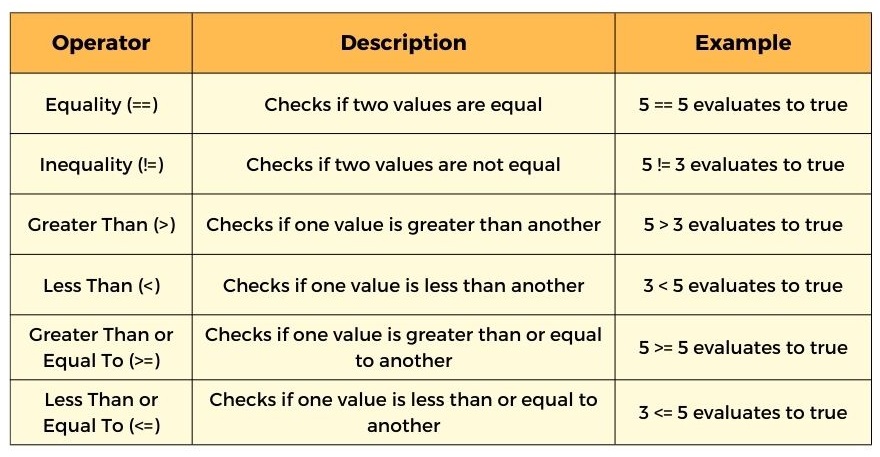
Example:
#include <stdio.h>
int main() {
int a = 5, b = 3;
// Equality (==)
if (a == b) {
printf("a is equal to b\n");
} else {
printf("a is not equal to b\n");
}
// Inequality (!=)
if (a != b) {
printf("a is not equal to b\n");
} else {
printf("a is equal to b\n");
}
// Greater Than (>)
if (a > b) {
printf("a is greater than b\n");
} else {
printf("a is not greater than b\n");
}
// Less Than (<)
if (a < b) {
printf("a is less than b\n");
} else {
printf("a is not less than b\n");
}
// Greater Than or Equal To (>=)
if (a >= b) {
printf("a is greater than or equal to b\n");
} else {
printf("a is not greater than or equal to b\n");
}
// Less Than or Equal To (<=)
if (a <= b) {
printf("a is less than or equal to b\n");
} else {
printf("a is not less than or equal to b\n");
}
return 0;
}
Output:
a is not equal to b
a is not equal to b
a is greater than b
a is not less than b
a is greater than or equal to b
a is not less than or equal to b
Logical Operators
Logical operators in C are used to perform logical operations on Boolean values (true or false). These operators allow you to combine or manipulate Boolean expressions.
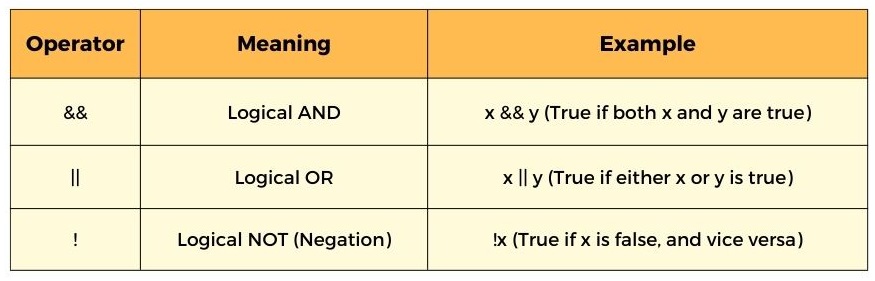
Example:
#include <stdio.h>
int main() {
int x = 5;
int y = 10;
int z = 3;
// Using logical AND (&&)
if (x > 0 && y > 0) {
printf("Both x and y are positive.\n");
} else {
printf("At least one of x and y is not positive.\n");
}
// Using logical OR (||)
if (x == 5 || z == 5) {
printf("Either x or z is equal to 5.\n");
} else {
printf("Neither x nor z is equal to 5.\n");
}
// Using logical NOT (!)
if (!(y == 10)) {
printf("y is not equal to 10.\n");
} else {
printf("y is equal to 10.\n");
}
return 0;
}
Output:
Both x and y are positive.
Either x or z is equal to 5.
y is equal to 10.
Explanation:
- In the first if statement,
x > 0 && y > 0
, both conditions must be true for the code inside the if block to execute. Since both x and y are positive, the condition evaluates to true. - In the second if statement,
x == 5 || z == 5
, at least one of the conditions must be true for the code inside the if block to execute. Since x is equal to 5, the condition evaluates to true. - In the third if statement,
!(y == 10)
, the logical NOT operator reverses the result of the condition(y == 10)
. Since y is equal to 10, the condition evaluates to false, and the NOT operator makes it true.
Assignment Operators
Assignment operators are fundamental in C programming for assigning values to variables. They come in two main forms: simple assignment and compound assignment.
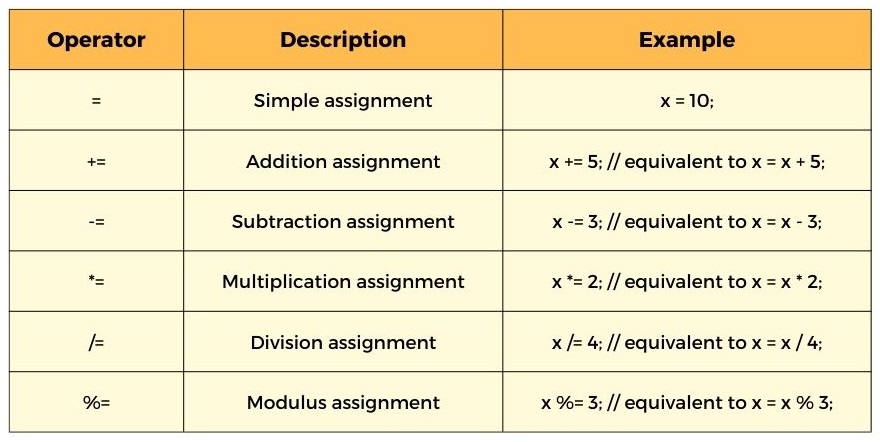
Explanation:
Simple assignment (=): Assigns the value on the right to the variable on the left.
Compound assignments (e.g., +=, -=, *=): Combines an arithmetic operation and an assignment. It executes the arithmetic operation and then stores the result in the variable on the left side.
Example:
#include <stdio.h>
int main() {
int x = 10;
// Simple assignment
int y = x;
printf("Value of y after simple assignment: %d\n", y);
// Compound assignment examples
x += 5; // Equivalent to x = x + 5;
printf("Value of x after addition assignment: %d\n", x);
x -= 3; // Equivalent to x = x - 3;
printf("Value of x after subtraction assignment: %d\n", x);
x *= 2; // Equivalent to x = x * 2;
printf("Value of x after multiplication assignment: %d\n", x);
x /= 4; // Equivalent to x = x / 4;
printf("Value of x after division assignment: %d\n", x);
x %= 3; // Equivalent to x = x % 3;
printf("Value of x after modulus assignment: %d\n", x);
return 0;
}
Output:
Value of y after simple assignment: 10
Value of x after addition assignment: 15
Value of x after subtraction assignment: 12
Value of x after multiplication assignment: 24
Value of x after division assignment: 6
Value of x after modulus assignment: 0
Increment and Decrement Operators
The increment (++) and decrement (–) operators in C programming are used to increment or decreases a variable’s value by 1. These operators are used in two ways: pre-increment/decrement and post-increment/decrement.
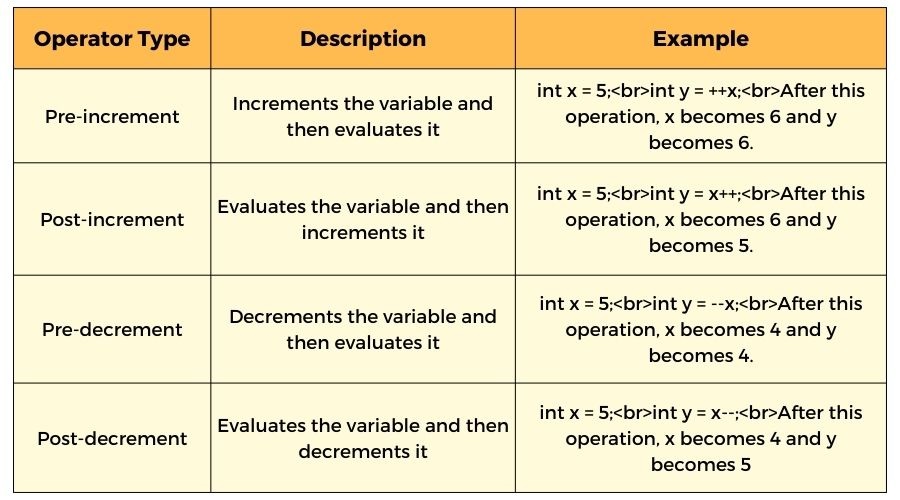
Example:
#include <stdio.h>
int main() {
int x = 5;
int y;
y = ++x; // Pre-increment
printf("Pre-increment: x = %d, y = %d\n", x, y);
x = 5;
y = x++; // Post-increment
printf("Post-increment: x = %d, y = %d\n", x, y);
x = 5;
y = --x; // Pre-decrement
printf("Pre-decrement: x = %d, y = %d\n", x, y);
x = 5;
y = x--; // Post-decrement
printf("Post-decrement: x = %d, y = %d\n", x, y);
return 0;
}
Output:
Pre-increment: x = 6, y = 6
Post-increment: x = 6, y = 5
Pre-decrement: x = 4, y = 4
Post-decrement: x = 4, y = 5
Bitwise Operators
Bitwise operators are operators in programming languages, including C, that manipulate individual bits of binary representations of numbers. Unlike arithmetic operators that operate on entire numbers, bitwise operators work on each bit of the binary representation independently.

Example:
#include <stdio.h>
int main() {
int a = 10; // Binary: 1010
int b = 12; // Binary: 1100
// Bitwise AND (&) operation
int result_and = a & b;
printf("Bitwise AND result: %d\n", result_and); // Output: 8 (binary: 1000)
// Bitwise OR (|) operation
int result_or = a | b;
printf("Bitwise OR result: %d\n", result_or); // Output: 14 (binary: 1110)
// Bitwise XOR (^) operation
int result_xor = a ^ b;
printf("Bitwise XOR result: %d\n", result_xor); // Output: 6 (binary: 0110)
// Left Shift (<<) operation
int result_left_shift = a << 2;
printf("Left Shift result: %d\n", result_left_shift); // Output: 40 (binary: 101000)
// Right Shift (>>) operation
int result_right_shift = a >> 2;
printf("Right Shift result: %d\n", result_right_shift); // Output: 2 (binary: 10)
// Ones' Complement (~) operation
int result_complement = ~a;
printf("Ones' Complement result: %d\n", result_complement); // Output: -11 (binary: 11110101)
return 0;
}
Conditional Operator (Ternary Operator)
Syntax:
condition ? expression1 : expression2
Usage:
- The conditional operator, often known as the ternary operator, is a simple technique to express conditional statements in C programming.
- It consists of three operands: a condition, a question mark (?), and two expressions separated by a colon.
- If the condition is true, expression1 is evaluated; otherwise, expression2 is evaluated.
- The conditional operator returns the value of expression1 or expression2, depending on whether the condition is true.
Example:
int num = 10;
int result;
// Using if-else statement
if (num > 5)
result = 1;
else
result = 0;
// Equivalent using conditional operator
result = (num > 5) ? 1 : 0;
In this example, if num
is greater than 5, result
will be assigned the value 1; otherwise, it will be assigned the value 0. The same logic is expressed using both an if-else statement and the conditional operator.
Miscellaneous Operators
Sizeof Operator
The sizeof operator in C is used to calculate the size of a variable or data type in bytes. It returns the size of the operand, which may be a variable, constant, or data type. This operator is very useful when allocating memory and working with arrays and structures.
Syntax:
sizeof(expression)
Example:
#include <stdio.h>
int main() {
int a;
double b;
char c;
printf("Size of int: %zu bytes\n", sizeof(a));
printf("Size of double: %zu bytes\n", sizeof(b));
printf("Size of char: %zu byte\n", sizeof(c));
return 0;
}
Also Read: Number based C Programs for Beginners
Comma Operator
The comma operator in C allows for the sequential evaluation of several expressions. It is frequently used when multiple expressions must be executed, but only the result of the last expression is considered. The comma operator has the least precedence of all C operators.
Syntax:
expression1, expression2
Example:
#include <stdio.h>
int main() {
int a = 1, b = 2, c = 3;
// Multiple expressions separated by comma
printf("a = %d, b = %d, c = %d\n", a, b, c), a++, b++, c++;
printf("After incrementing: a = %d, b = %d, c = %d\n", a, b, c);
return 0;
}
In this example, the comma operator is used to increment variables a
, b
, and c
sequentially, and the result of the last expression (c++
) is considered as the result of the overall expression.